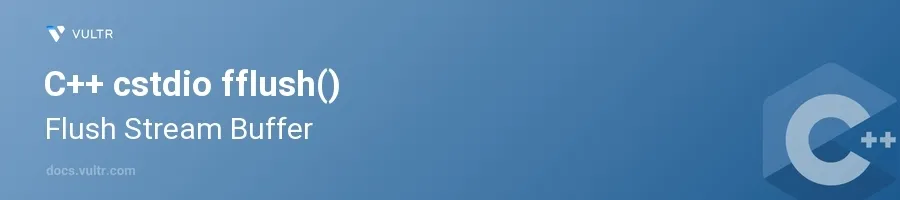
Introduction
The fflush()
function in C++ is a standard library function found within the <cstdio>
header, utilized primarily to flush a stream's output buffer. This ensures that all buffered data is written to the respective output stream, such as a file or console, which is critical in scenarios where precise timing and sequence of output is necessary.
In this article, you will learn how to effectively use the fflush()
function in various programming scenarios. Discover how to ensure data consistency and avoid potential i/o errors or delays in program execution with proper buffer management.
Understanding fflush()
Basics of fflush()
Recognize that
fflush()
is used to clear (or flush) the output buffer of a stream.Apply
fflush()
to any output stream to send data immediately to the file or terminal.cpp#include <cstdio> int main() { printf("Buffering..."); fflush(stdout); }
Here,
fflush(stdout)
ensures that the text "Buffering..." is immediately displayed on the console, rather than waiting for the buffer to fill up or for the program to terminate.
Common Use Cases
Employ
fflush()
in logging mechanisms to make sure all messages are logged instantly, particularly during debugging sessions.Use within server applications to deliver output updates in real-time.
cppfprintf(stderr, "Error encountered. Flushing buffer now.\n"); fflush(stderr);
In this snippet,
fflush(stderr)
immediately writes the error message to the standard error stream, which is useful in real-time monitoring of application health.
Advanced Usage of fflush()
Impact on Different Stream Types
Understand that using
fflush()
on input streams is undefined behavior, except for theNULL
pointer which flushes all output streams.Use carefully in file streams to ensure data integrity and avoid excessive disk operations, as it might impact performance.
cppFILE *file = fopen("example.txt", "w"); fputs("Writing to file...", file); fflush(file); fclose(file);
This code writes a message to a file and flushes the buffer with
fflush(file)
, which is pivotal in applications where immediate file updates are necessary.
Error Handling
Check the return value of
fflush()
.Implement error handling mechanisms if
fflush()
returns EOF.cppif (fflush(file) == EOF) { perror("Failed to flush the file stream!"); }
If
fflush()
fails, it returns EOF. Handling such cases ensures robustness, especially when dealing with critical file operations.
Conclusion
The fflush()
function in C++ plays a vital role in managing output buffers, ensuring that data is written out promptly and consistently. From enhancing debugging capabilities to real-time system logging, proper use of fflush()
can significantly improve program reliability and performance. By incorporating the discussed techniques, you can effectively manage data outputs in your applications to avoid unnecessary delays and errors.
No comments yet.