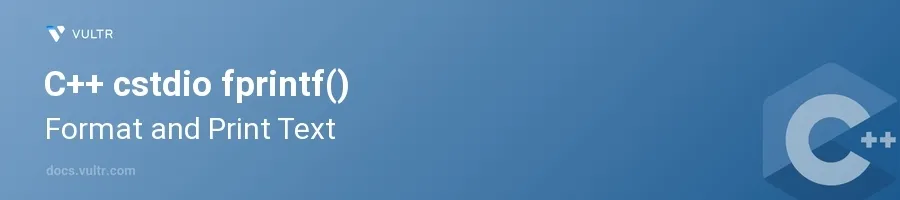
Introduction
The C++ fprintf()
function, provided by the cstdio
library, is an essential tool for formatted output to a file or stream. This function is widely used in both simple and complex file operations, especially when precise control over text format and output destination is required. It's beneficial for tasks such as logging, saving formatted data, or creating custom file outputs in C++ applications.
In this article, you will learn how to effectively harness the fprintf()
function to format and print text to various streams. Discover the syntax, different format specifiers, and practical usage examples to master file output operations in your C++ projects.
Understanding fprintf() Syntax and Specifiers
Syntax of fprintf()
Recognize the importance of understanding the correct syntax:
The general syntax of
fprintf()
is:cppint fprintf(FILE *stream, const char *format, ...);
The function returns the number of characters that are printed or a negative value if an error occurs.
Common Format Specifiers
Familiarize yourself with different format specifiers used in
fprintf()
:%d
or%i
- for integers%f
- for floating-point numbers%s
- for strings%c
- for single characters%x
or%X
- for hexadecimal representation
Each specifier allows formatting of different data types to the destined output stream.
Practical Examples Using fprintf()
Printing to a File
Understand how to direct output to a file rather than the console:
First, ensure you have a pointer to a
FILE
object, typically obtained via thefopen()
function:Use
fprintf()
to write formatted text to the file.cppFILE* filePtr = fopen("output.txt", "w"); if (filePtr == nullptr) { perror("Failed to open file"); } else { fprintf(filePtr, "Hello, %s. Your score is %d.\n", "Alice", 95); fclose(filePtr); }
Here,
fprintf()
writes a formatted string to "output.txt", including a name and an integer score, then closes the file.
Formatting Numbers
Use
fprintf()
to handle numeric data with precision specifications:Format floating-point numbers using precision specifiers:
cppFILE* filePtr = fopen("output.txt", "a"); // Appends to the file if (filePtr == nullptr) { perror("Failed to open file"); } else { fprintf(filePtr, "Pi is approximately %.2f\n", 3.14159); fclose(filePtr); }
This example formats the value of Pi to two decimal places in "output.txt".
Handling Multiple Data Types
Combine various data types in a single
fprintf()
statement:Format output that includes strings, integers, and floats together:
cppFILE* filePtr = fopen("data.txt", "w"); if (filePtr == nullptr) { perror("Failed to open file"); } else { fprintf(filePtr, "%s %d %0.2f\n", "Data:", 100, 123.456); fclose(filePtr); }
The example demonstrates how to write mixed data to a file, offering flexibility in data management.
Conclusion
The fprintf()
function in C++ is a powerful utility for formatted file output, allowing detailed control over how data is presented in files. By mastering the use of fprintf()
with various data types and format specifiers, you enhance the capability to produce structured and readable files efficiently. Implement these techniques in your C++ projects for effective data logging, file saving, and much more, ensuring your application's output is both functional and professional.
No comments yet.