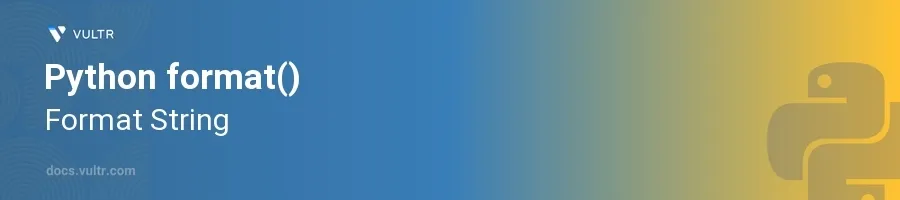
Introduction
The format()
function in Python is integral for creating formatted strings, especially useful in situations where you need to construct text with variable data in a readable and aesthetically pleasing way. This function provides a wide array of formatting options, allowing for alignment, padding, precision, and much more, simplifying the process of working with strings.
In this article, you will learn how to effectively use the format()
function to manipulate and display strings aesthetically. Uncover the benefits of using various format specifications for different data types and how to incorporate these techniques into your Python programming.
Basic Usage of format()
Insert Variables into Strings
Start with a simple string that has placeholders defined by curly braces
{}
.Use the
format()
function to substitute the placeholders with variable values.pythonuser_name = "Alice" user_age = 30 formatted_string = "Name: {}, Age: {}".format(user_name, user_age) print(formatted_string)
This code snippet replaces
{}
with theuser_name
anduser_age
variables respectively. The output would beName: Alice, Age: 30
.
Formatting Numbers for Consistency
Define a number that you need to display to a certain number of decimal places.
Use
format()
to control the precision of the number.pythonpi_value = 3.14159265 output = "Formatted Pi: {:.2f}".format(pi_value) print(output)
In this example,
:.2f
specifies that the floating-point number (pi_value
) should be formatted to two decimal places. The result isFormatted Pi: 3.14
.
Padding and Aligning Strings
Determine the width of the field in which the string should fit.
Use
format()
to align the text and to add padding characters.pythonpadded_string = "{:>10}".format("test") print(padded_string)
Here,
:>10
directs that the stringtest
should be right-aligned within a field 10 characters wide. It fills the remaining space with spaces on the left, producingtest
.
Advanced Formatting Techniques
Named Placeholders
Create a string that uses named placeholders instead of empty braces.
Pass the variable values by name into the
format()
function.pythondata_string = "Name: {name}, Age: {age}".format(name="Bob", age=40) print(data_string)
Utilizing named placeholders like
{name}
and{age}
enhances readability and maintainability, especially in strings involving multiple variables. The output would beName: Bob, Age: 40
.
Formatting With Dictionaries
Store data in a dictionary where keys match the placeholders in the string.
Use the
**
operator to unpack the dictionary directly into theformat()
function.pythonuser_info = {'name': 'Carol', 'age': 35} info_string = "Name: {name}, Age: {age}".format(**user_info) print(info_string)
This method simplifies passing multiple variable names, reducing potential errors and improving code clarity. The result is
Name: Carol, Age: 35
.
Conclusion
The format()
function in Python stands as a powerful tool for string manipulation, aiding in presenting data cleanly and consistently across various applications. From simple replacements to complex alignments and precision control, the function's versatility in handling strings is indispensable in creating readable and well-formatted output. Apply these formatting techniques to enhance the presentation layer of your Python applications, ensuring the data not only communicates effectively but also looks appealing.
No comments yet.