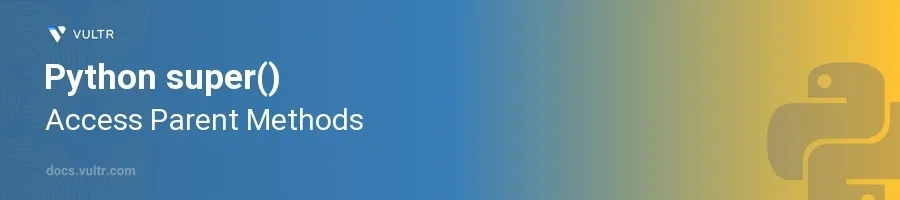
Introduction
The super()
function in Python is a built-in function that allows you to call methods of a superclass from a subclass. This function plays a crucial role in facilitating cooperative multiple inheritance and is often used when extending the functionality of inherited methods. It enables a framework where subclasses can alter or extend the behavior of superclass methods without directly modifying the original method implementations.
In this article, you will learn how to effectively use the super()
function in object-oriented programming with Python. Explore the use of super()
in single inheritance and multiple inheritance scenarios, and discover how it helps in accessing overridden methods of parent classes.
Using super() in Single Inheritance
Access Parent Class Methods
Define a parent class with a method.
Create a subclass that inherits from this parent class.
Use
super()
in the subclass to access the parent class method.pythonclass Parent: def show(self): print("Inside Parent") class Child(Parent): def display(self): print("Inside Child") super().show() obj = Child() obj.display()
This code defines two classes,
Parent
andChild
, whereChild
inherits fromParent
. Theshow()
method in theParent
class is called from thedisplay()
method of theChild
class usingsuper()
. The output will be "Inside Child" followed by "Inside Parent".
Override and Extend Parent Class Methods
Override a method in the subclass that already exists in the parent class.
Use
super()
to enhance, rather than replace, the parent class method functionality.pythonclass Parent: def show(self): print("Parent method") class Child(Parent): def show(self): print("Child method before calling super()") super().show() print("Child method after calling super()") obj = Child() obj.show()
In this snippet, the
show()
method in theChild
class first prints a message, then calls the same method from theParent
class usingsuper()
, and finally prints another message. This results in a sequence of prints that demonstrates extending the functionality of the parent method.
Using super() in Multiple Inheritance
Access Methods from Multiple Parents
Define two or more parent classes with unique methods.
Create a subclass that inherits from these multiple parent classes.
Use
super()
to access methods from multiple parent classes sequentially.pythonclass Father: def activity(self): print("Father's activity") class Mother: def activity(self): print("Mother's activity") class Child(Father, Mother): def activity(self): print("Child's activity") super().activity() obj = Child() obj.activity()
In this example, both
Father
andMother
classes have a method calledactivity()
. TheChild
class, which inherits from both, overrides theactivity()
method and usessuper()
to call the method from the first superclass listed in the inheritance tuple (Father
in this case), thus demonstrating howsuper()
resolves method calls in multiple inheritance.
Conclusion
The super()
function in Python is essential for invoking superclass methods effectively, especially in complex class hierarchies involving multiple inheritance. It eliminates the need for explicitly referencing parent classes, making the code more maintainable and less prone to errors. By mastering super()
, you ensure your code is robust, readable, and follows good object-oriented design principles. Whether in single or multiple inheritance scenarios, super()
can simplify method calls and help manage method overriding systematically.
No comments yet.