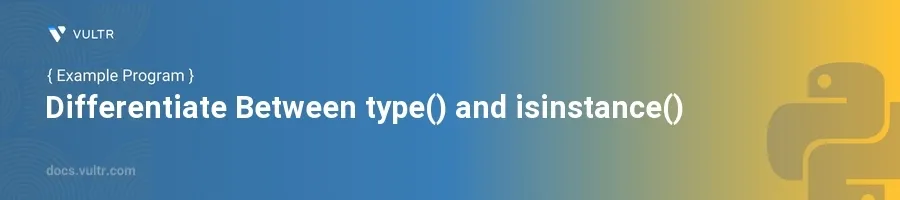
Introduction
Understanding the differences between the type()
function and the isinstance()
function in Python is crucial for effective type checking and writing more adaptable, maintainable code. While both are used to check the type of variables, they each serve unique roles and are appropriate in different scenarios.
In this article, you will learn how to distinguish and appropriately use type()
and isinstance()
through practical examples. This knowledge will aid in enhancing flexibility in handling polymorphism and ensuring that the guidelines of Python's dynamic typing are met efficiently.
Using type()
type()
is used to obtain the type of the given object. This function is straightforward and is often used when you only need to know the exact type of an object.
Example to Demonstrate type()
Define a variable with a specific data type.
Use the
type()
function to check the type of the variable.pythonmy_var = 10 print(type(my_var))
This code will output
<class 'int'>
, indicating thatmy_var
is an integer.
Limitations of type()
- Fails to recognize subclasses as an instance of a parent class.
- Suitable when exact type checking (without considering inheritance) is required.
Using isinstance()
isinstance()
checks if an object is an instance of a class or a tuple of classes. It's more flexible than type()
because it supports class inheritance, making it ideal in many object-oriented programming scenarios.
Example to Demonstrate isinstance()
Define a class and a subclass.
Create an instance of the subclass.
Use
isinstance()
to check if the object is an instance of the parent class.pythonclass Parent: pass class Child(Parent): pass child_instance = Child() print(isinstance(child_instance, Parent))
This code will output
True
, demonstrating thatchild_instance
is recognized as an instance of bothChild
and its parent classParent
.
Benefits of isinstance()
- Acknowledges class inheritance.
- More flexible in checking types, which is useful in polymorphism.
Comparative Study Through Examples
Understanding when to use type()
vs isinstance()
can be highlighted by a side-by-side comparison:
Create a class and subclass as before.
Compare the behavior of
type()
andisinstance()
with an instance of the subclass.pythonclass Base: pass class Derived(Base): pass derived_instance = Derived() # Using type() print(type(derived_instance) == Base) # Outputs: False # Using isinstance() print(isinstance(derived_instance, Base)) # Outputs: True
Here,
type()
checks for exact type matching, whileisinstance()
considers the inheritance chain.
Conclusion
Choosing between type()
and isinstance()
depends largely on the specific requirements of your program. Use type()
when you need to assert exact type equality without regard for inheritance. Opt for isinstance()
when dealing with object-oriented designs where an object's ability to behave as an instance of its parent class (or classes) is necessary. By utilizing these functions appropriately, you ensure the robustness and flexibility of your Python code.
No comments yet.