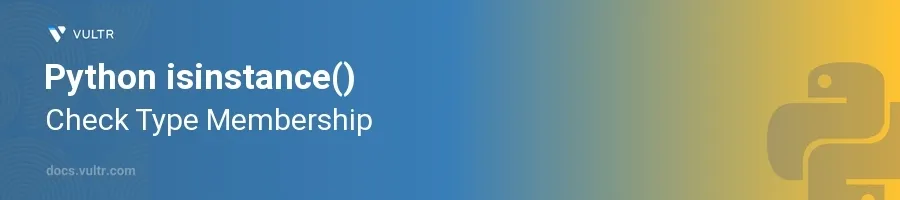
Introduction
The isinstance()
function in Python serves as a crucial tool for checking whether an object is an instance of a particular class or a tuple of classes. This is particularly useful in ensuring that variables conform to expected types, thereby enhancing the robustness and reliability of the code by catching type errors early in the development process.
In this article, you will learn how to utilize the isinstance()
function effectively across various data types and scenarios. Explore how to implement type checks for both single and multiple types, and see how this function can be integrated within functions to validate input types.
Checking Single Type Membership
Verify if an Object is of a Specific Type
Create an object of a specific data type.
Use
isinstance()
to confirm if the object belongs to the type you're checking.pythonnumber = 10 print(isinstance(number, int)) # Checking if 'number' is an instance of 'int'
This code snippet checks whether
number
is an instance of theint
type. It returnsTrue
sincenumber
is indeed an integer.
Handling Multiple Object Instances
Create different objects of various types.
Apply
isinstance()
to each object to validate its type.pythontext = "Hello" print(isinstance(text, str)) # Validating if 'text' is a string
Here,
isinstance()
checks iftext
is a string (str), returningTrue
astext
is a string.
Checking Multiple Types Membership
Determine if an Object Belongs to One of Several Types
Initiate an object of a specific data type.
Use
isinstance()
with a tuple for multiple type checks.pythoninput_data = 3.14 print(isinstance(input_data, (int, float, complex))) # Checks if 'input_data' is either int, float, or complex
In this snippet,
isinstance()
evaluates whetherinput_data
is an instance of any of the types in the tuple (int, float, complex). Sinceinput_data
is a float, it returnsTrue
.
Using isinstance() in Conditional Statements
Use
isinstance()
within an if statement to execute code based on type confirmation.pythondef process_data(data): if isinstance(data, (list, tuple)): return sum(data) # Only execute if 'data' is a list or tuple else: return "Invalid type" print(process_data([1, 2, 3]))
This function,
process_data
, checks ifdata
is either a list or a tuple before attempting to sum the elements. It returns the sum if the check passes, otherwise, it returns an "Invalid type" message.
Conclusion
The isinstance()
function in Python is invaluable for ensuring type correctness in data handling and processing scenarios. By incorporating type checks using isinstance()
, you improve the safety and predictability of your Python programs. Employ this function to verify single or multiple type memberships and integrate type validation seamlessly into your function logic, ensuring accurate operations based on data types. Use the described techniques to enhance your programming practices by enforcing type specificity where necessary.
No comments yet.