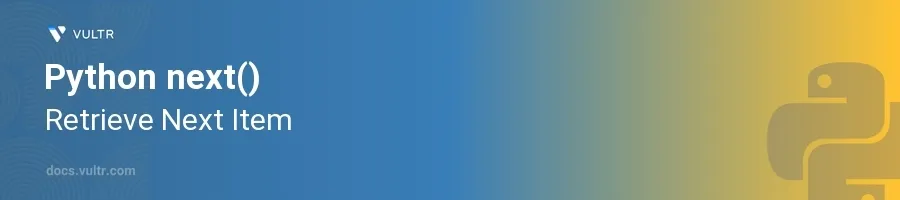
Introduction
The next()
function in Python is a built-in utility that retrieves the next item from an iterator. It’s particularly useful when you need to access elements from an iterable object, such as lists or generators, one at a time without using a loop. This function simplifies control flow and is often used in situations where operations on items terminate on specific conditions.
In this article, you will learn how to use the next()
function effectively across different scenarios. Explore how this function works with various types of iterables, handles default values, and interacts with the StopIteration
exception.
Using next() with Lists and Generators
Retrieve Elements Sequentially
Convert a list to an iterator using the
iter()
function.Use
next()
to fetch the next available item.pythonfruits = iter(['apple', 'banana', 'cherry']) print(next(fruits)) print(next(fruits))
The first
next(fruits)
call retrieves 'apple', and the second call retrieves 'banana'. Each call tonext()
moves the iterator to the next item.
Handling StopIteration Exception
Understand that
next()
raises aStopIteration
when no items are left.Handle this exception appropriately to avoid runtime errors.
pythonfruits = iter(['apple']) print(next(fruits)) # Outputs 'apple' try: print(next(fruits)) except StopIteration: print("No more items.")
After printing 'apple', attempting to retrieve another item leads to a
StopIteration
which is caught by theexcept
block, outputting "No more items."
Using next() with a Default Value
Provide a default value to
next()
to return when the iterator is exhausted.This prevents
StopIteration
and provides a fallback value.pythonfruits = iter(['apple']) print(next(fruits, 'No more fruits')) print(next(fruits, 'No more fruits'))
This will print 'apple' and then 'No more fruits', as the default value is returned when no items are left.
Working with next() and Custom Iterators
Implementing a Simple Iterator Class
Define a class with special methods
__iter__()
and__next__()
.Use this custom iterator with
next()
to fetch elements.pythonclass CountDown: def __init__(self, start): self.current = start def __iter__(self): return self def __next__(self): if self.current == 0: raise StopIteration self.current -= 1 return self.current countdown = iter(CountDown(3)) print(next(countdown)) print(next(countdown)) print(next(countdown))
The
CountDown
class counts down from a starting number to zero. Thenext()
function calls retrieve subsequent numbers until the counter reaches zero andStopIteration
is raised.
Advanced Usage of next()
Cycling Through Items Infinitely
Utilize Python’s
itertools.cycle
to create a repeating iterator.Use
next()
to cycle through the items continuously.pythonimport itertools colors = itertools.cycle(['red', 'green', 'blue']) print(next(colors)) print(next(colors)) print(next(colors)) print(next(colors)) # Starts over with 'red'
The output will cycle through 'red', 'green', 'blue', and then return to 'red' again, demonstrating infinite cycling.
Conclusion
The next()
function in Python offers a streamlined approach to iterating through an iterable without explicitly using a loop, making code cleaner and more expressive. By utilizing next()
to handle iterators, manage default values, and integrate with custom and infinite iterators, you enhance the flexibility and control of data access patterns in your code. Implement these techniques to improve both the performance and readability of your Python scripts.
No comments yet.