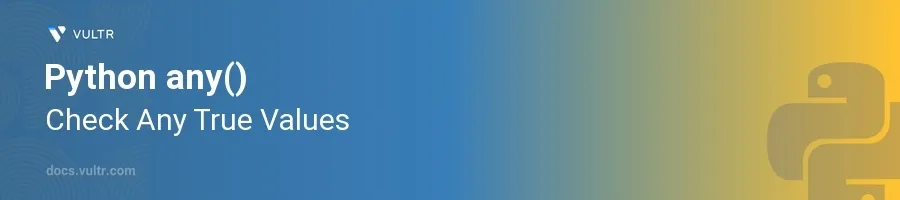
Introduction
The any()
function in Python checks if any element in an iterable is True
. This function is extremely useful when you need a quick way to evaluate if at least one of the conditions in a composite query returns True
. It's commonly used in decision-making processes within programs, particularly in data analysis and filtering operations.
In this article, you will learn how to effectively utilize the any()
function in various programming scenarios. Discover how this function can streamline code when working with lists, dictionaries, and other iterable objects while exploring its behavior with different data types.
Using any() with Lists
Check if Any Element is True
Create a list of boolean values.
Use the
any()
function to evaluate the list.pythonbool_list = [False, False, True, False] result = any(bool_list) print(result)
This code checks the list
bool_list
for anyTrue
values. Since there is oneTrue
value in the list,any()
returnsTrue
.
Working with Numeric Values
Realize that non-zero numbers are treated as
True
in Python.Define a list with numeric values where at least one is non-zero.
Apply the
any()
function.pythonnum_list = [0, 0, 1, 0] result = any(num_list) print(result)
Here,
any()
evaluates each number innum_list
, and since1
is equivalent toTrue
, it outputsTrue
.
Using any() with Composite Conditions
Create a list that contains multiple boolean expressions.
Use
any()
to see if any of the conditions areTrue
.pythonx = 5 conditions = [x > 10, x == 5, x % 2 == 0] result = any(conditions) print(result)
In this snippet, the three conditions evaluate to
False
,True
, andFalse
, respectively. Theany()
function finds theTrue
value and thus returnsTrue
.
Using any() with Dictionaries
Check if Any Key or Value Matches a Condition
Understand that evaluating a dictionary directly with
any()
checks keys by default.Create a dictionary where you look for any specific condition in the keys or values.
Apply the
any()
function appropriately.pythondict_data = {'a': 0, 'b': False, 'c': 20} key_check = any(key == 'b' for key in dict_data) value_check = any(value > 0 for value in dict_data.values()) print("Any key matched: ", key_check) print("Any value positive: ", value_check)
In the example,
key_check
verifies if 'b' is a key indict_data
, andvalue_check
tests for any positive values in the dictionary.
Conclusion
The any()
function in Python is a powerful tool for quickly checking the presence of a True
condition within an iterable. Its ability to abstract complex conditions into a single line of code enhances readability and efficiency in Python scripts. Use this function in various situations, from simple list checks to complex dictionary evaluations, to simplify your decision-making code structures. By implementing the techniques discussed, you ensure your code remains clean, readable, and efficient.
No comments yet.