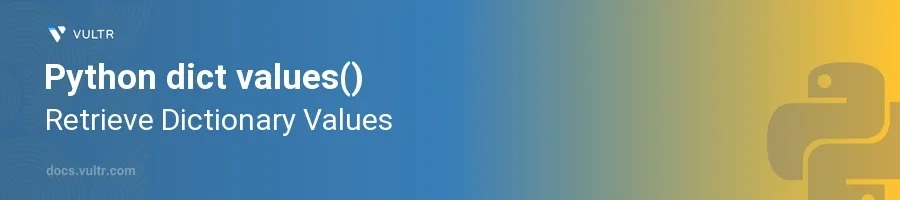
Introduction
The values()
method in Python is essential for accessing the values in a dictionary. This method returns a view object that displays a list of all the values set in the dictionary. This is particularly useful when you need to examine, modify, or operate on all the values in a dictionary without needing to handle the keys.
In this article, you will learn how to effectively use the values()
method to access and manipulate dictionary values in Python. Explore practical examples that demonstrate how to iterate over values, perform operations with them, and combine them with other programming constructs for efficient data handling.
Retrieving Values from a Dictionary
Basic Retrieval of All Values
Create a dictionary with some key-value pairs.
Use the
values()
method to retrieve the values.pythonmy_dict = {'apple': 3, 'banana': 5, 'cherry': 8} values = my_dict.values() print(values)
This code prints the values from
my_dict
which are3, 5, 8
. The output is a view object which reflects any changes to the dictionary.
Iterating Over Values
With the values retrieved, iterate over them using a simple loop.
pythonfor value in my_dict.values(): print(value)
This loop prints each value individually, allowing operations to be performed directly on each value.
Operations Using Dictionary Values
Summing All Values
Retrieve and sum all the values in a dictionary using the
sum()
function.pythontotal_fruit = sum(my_dict.values()) print(total_fruit)
Summing the values directly, outputs the total count of fruits in
my_dict
, i.e.,16
.
Conditional Operations on Values
Use conditional statements within a list comprehension to perform filtered operations.
pythonhigh_count = [value for value in my_dict.values() if value > 4] print(high_count)
This code analyzes the values and prints only those greater than 4. Here, the output will be
[5, 8]
.
Conclusion
The values()
method in Python is a direct and efficient way to access all the values from a dictionary. It's an indispensable tool for scenarios where only values matter and not their associated keys. By leveraging this functionality, easily perform operations like summing up values, iterating for specific conditions, and more. With the techniques discussed, you enhance your dictionary data handling skills, ensuring smooth and efficient programming practices.
No comments yet.