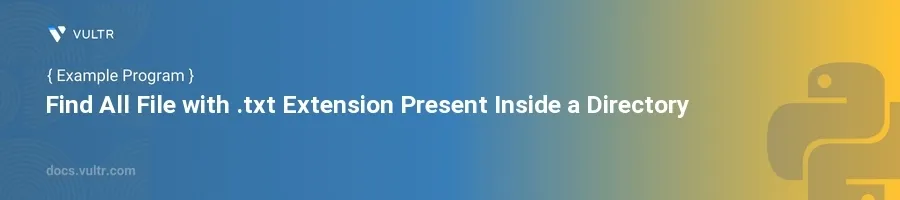
Introduction
Working with files in Python allows for robust file manipulation and management, including recognizing and organizing files according to their extensions. A common task you might face as a developer or system administrator is identifying all .txt
files within a specific directory. This is particularly useful for tasks like batch processing, data analysis, or log file management.
In this article, you will learn how to create a Python program to find all files with a .txt
extension in a given directory. Explore different methods to accomplish this task efficiently using Python's built-in libraries.
Utilizing the os Module
Basic Directory Scanning for '.txt' Files
Import the
os
module, which provides a way to work with file system paths, directories, and file names.Use
os.listdir()
to fetch all entries in the specified directory.Filter the entries to find files that end with the '.txt' extension.
pythonimport os directory = '/path/to/directory' files = os.listdir(directory) txt_files = [file for file in files if file.endswith('.txt')] for file in txt_files: print(file)
This code block lists all the
.txt
files in the specified directory. Thelistdir()
method retrieves all files and directories in the provided path, and the list comprehension filters out files ending with '.txt'.
Combining Paths Properly
It's essential to handle file paths appropriately across different operating systems.
Use
os.path.join()
to construct the full file path correctly.pythonpath = '/path/to/directory' txt_files = [file for file in os.listdir(path) if file.endswith('.txt')] for file in txt_files: full_path = os.path.join(path, file) print(full_path)
This enhancement ensures that the file paths are correctly formed by joining the directory path with the file name efficiently.
Using the glob Module for Pattern Matching
Simple Globbing to Find '.txt' Files
Use the
glob
module which provides a functionglob.glob()
specifically designed to find all pathnames matching a specified pattern.Direct the
glob()
function to match.txt
files within the target directory.pythonimport glob directory = '/path/to/directory' txt_files = glob.glob(f'{directory}/*.txt') for file in txt_files: print(file)
This code directly fetches all files that match the '*.txt' pattern in the specified directory. The
glob()
function simplifies the process by ignoring non-matching files entirely.
Conclusion
Leverage Python's os
and glob
modules to find all files with a .txt
extension in a directory efficiently. This skill is essential for file management tasks in many professional scenarios. Whether for simple file sorting or complex file manipulation programs, understanding these methods ensures you can handle files in Python effectively, enhancing productivity and organizational workflows.
No comments yet.