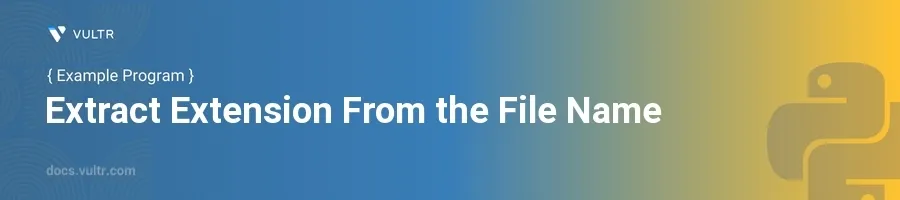
Introduction
Working with file names and extensions is a common task in many programming scenarios, especially when dealing with files in automation, data processing, or user interfaces. Understanding how to extract file extensions efficiently can help in managing various file types and implementing logic based on file formats.
In this article, you will learn how to efficiently extract file extensions from file names using Python. Dive into real-world examples that showcase simple and effective methods to accomplish this task, providing you with the skills to handle file extensions in your Python projects.
Extracting File Extensions Using Python's os
Module
Understanding the os.path.splitext
Method
Import the
os
moduleUse
os.path.splitext()
to split the file path.pythonimport os filename = "example.jpeg" root, ext = os.path.splitext(filename) print("File Extension:", ext)
This code splits the filename into the root and the extension. The
ext
variable contains the file extension,.jpeg
.
Handling File Names with Multiple Dots
Recognize that file names might contain dots other than the extension separator.
Apply
os.path.splitext()
to extract the extension correctly.pythonfilename = "archive.tar.gz" root, ext = os.path.splitext(filename) print("File Extension:", ext)
The result will show '.gz' as the extension. Note that if dealing with compound extensions like
.tar.gz
, additional logic would be needed to capture the entire extension.
Utilizing Python's pathlib
Module
Simple Extension Extraction with Path.suffix
Import the
pathlib
module.Use the
suffix
property on aPath
object to get the extension.pythonfrom pathlib import Path filename = Path("report.pdf") ext = filename.suffix print("File Extension:", ext)
Here,
ext
will be.pdf
. ThePath.suffix
property automatically handles the file extension.
Handling Files Without Extensions
Be aware that not all files have extensions.
Use the
suffix
property to safely handle such cases.pythonfilename = Path("README") ext = filename.suffix print("File Extension:", ext) # This will print an empty string
The code handles files without extensions gracefully, returning an empty string if no extension is present.
Conclusion
Extracting file extensions in Python can be efficiently handled using both the os
module and the pathlib
module. Depending on the complexity of your file handling needs and your preference for modern Python features, you can select the appropriate approach. The methods discussed ensure robust handling of various file name formats and provide a foundation for further file manipulation tasks in your Python applications. Adopt these techniques to ensure your programs can effectively interact with file systems and manage files based on their types.
No comments yet.