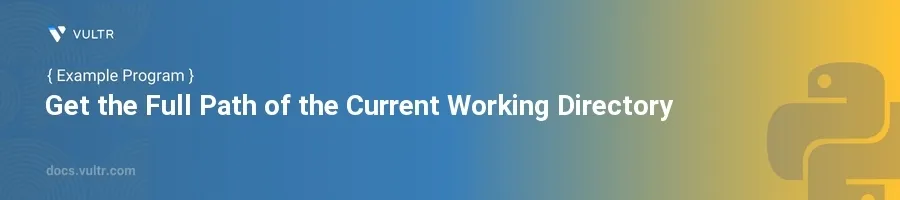
Introduction
Navigating file systems is a common task in programming, especially when your application needs to interact with files and directories. Python simplifies this through its robust standard libraries, making it easy to manage paths, check locations, and retrieve full directory paths. One such task is printing the full path of the current working directory, which is essential for file management and scripts that depend on file locations.
In this article, you'll learn how to print the current working directory in Python. We'll explore how to get the current directory path with os
and pathlib
, and how to use these tools to construct dynamic file paths. This knowledge will help you build more resilient and portable Python applications.
Basic Retrieval of the Current Working Directory
Using the os
Module
Import the
os
module, which provides a portable way of using operating system dependent functionality.Use the
os.getcwd()
function to get the current working directory.pythonimport os current_directory = os.getcwd() print("Current Working Directory:", current_directory)
This code imports the
os
module and uses thegetcwd()
function to print the current working directory. The output will reflect the absolute path of the directory from where the Python script is executed.
Understanding the Output
- The output prints the absolute path to the current working directory from which the Python script runs.
- This method ensures that you always know the execution context of your script, which is crucial for handling relative paths.
Advanced Usage with pathlib
Leveraging the pathlib
Module for Modern Python
Import the
pathlib
module, which provides object-oriented filesystem paths.Use the
Path.cwd()
method frompathlib
to get the current working directory in a more modern and semantic way.pythonfrom pathlib import Path current_directory = Path.cwd() print("Current Working Directory:", current_directory)
The
Path.cwd()
method returns aPath
object representing the file system path of the current working directory.pathlib
provides a high-level interface for filesystem interactions, making this method preferable in modern Python code for its readability and ease of use.
Benefits of Using pathlib
- Provides an object-oriented interface to handle file and directory paths.
- Simplifies tasks like joining paths, checking if a file exists, and retrieving the path of the current file.
- Easily convertible to strings and vice versa, which makes it compatible with functions expecting string paths.
Combining Paths with Current Directory for File Operations
Creating and Managing File Paths
Use the path obtained from previous methods to manage and create file paths dynamically.
Combine path operations using
os.path.join
orpathlib.Path
operations for creating full file paths.python# Using os file_path = os.path.join(os.getcwd(), "example_file.txt") print("Full path to file:", file_path) # Using pathlib file_path = Path.cwd() / "example_file.txt" print("Full path to file using pathlib:", file_path)
Above,
os.path.join
and the division operator onpathlib.Path
objects are used to append "example_file.txt" to the current working directory. These methods ensure that file paths are built correctly across different operating systems by handling different path separators transparently.
Importance of Correct Path Handling
- Avoid common errors with file paths, such as incorrect separators or mixed path formats.
- Ensure your script is portable and works across different operating systems.
- Essential when you need to get the current file path in Python or handle application directories reliably.
Conclusion
Finding the current working directory in Python is a simple yet powerful capability. Whether you use os.getcwd()
for a string-based method or Path.cwd()
for a modern, object-oriented approach, Python offers versatile tools for handling file paths.
Understanding how to get the absolute path, check the working directory, and combine paths dynamically will make your applications more portable and error-resistant. Use these techniques to print the current working directory in Python, retrieve current path information, and get the full path of a file in your projects.
No comments yet.