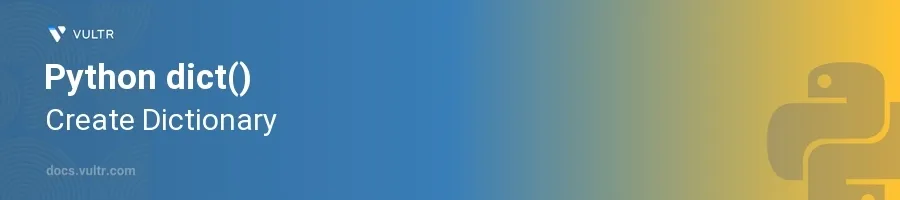
Introduction
The dict()
constructor in Python provides a versatile way of creating dictionaries from iterable key-value pairs or from keyword arguments. Often used for organizing data into a structured format, dictionaries are crucial for tasks that involve data manipulation and retrieval.
In this article, you will learn how to leverage the dict()
constructor in various scenarios to create dictionaries efficiently. Explore methods to initialize dictionaries using lists, tuples, and keyword arguments while understanding the syntax and behavior of the dict()
function.
Creating Dictionaries Using dict()
Initialize with Keyword Arguments
Utilize keyword arguments to directly pass keys and values.
Create a dictionary using simple syntax with
dict()
.pythonemployee = dict(name="John", age=30, department="Finance") print(employee)
This code creates a dictionary named
employee
with keys 'name', 'age', and 'department' and their corresponding values. The output shows the dictionary reflecting these key-value pairs.
Construct from a List of Tuples
Prepare a list of tuples where each tuple represents a key-value pair.
Convert this list into a dictionary.
pythonitems = [("key1", "value1"), ("key2", "value2")] dictionary = dict(items) print(dictionary)
In this snippet,
dict()
interprets each tuple in the listitems
as a key-value pair, transforming the list into a dictionary with keys 'key1' and 'key2'.
Convert Two Parallel Lists into a Dictionary
Use two parallel lists: one for keys, another for values.
Apply the
zip()
function followed by thedict()
constructor.pythonkeys = ["id", "name", "age"] values = [1, "Alice", 25] mapped_data = dict(zip(keys, values)) print(mapped_data)
Here,
zip(keys, values)
pairs keys and values from separate lists into tuples. Thedict()
then coverts these tuples into a dictionary representing an entry with 'id', 'name', and 'age' as keys.
Create from Keys with a Common Value
Use a list of keys and a default value to quickly initiate a dictionary.
Employ dictionary comprehension with
dict.fromkeys()
.pythonkeys = ["apple", "banana", "cherry"] default_value = 0 fruit_dict = dict.fromkeys(keys, default_value) print(fruit_dict)
dict.fromkeys()
sets the samedefault_value
to each key in thekeys
list, useful for initializing dictionaries with default values or counters.
Conclusion
The dict()
function in Python is a flexible and straightforward tool for dictionary creation, handling multiple data inputs like keyword arguments or lists of tuples. The techniques discussed enable you to structure data efficiently, whether starting from scratch or transforming existing collections. Embrace these approaches to enhance data handling and organization in your Python scripts.
No comments yet.