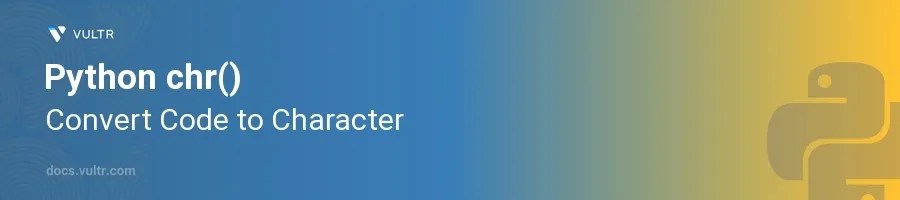
Introduction
The chr()
function in Python is invaluable when you need to convert an integer representing a Unicode code point into its corresponding character. This is especially helpful in situations involving data encoding, decoding, or when handling character transformations in various applications like text processing or generation.
In this article, you will learn how to effectively use the chr()
function across different scenarios. Explore its application in basic character conversion, error handling when invalid Unicode code points are used, and in generating sequences of characters.
Basic Usage of chr()
Convert an Integer to a Character
Ensure the integer represents a valid Unicode code point.
Use the
chr()
function to convert the integer.pythonunicode_point = 65 character = chr(unicode_point) print(character)
This code converts the Unicode code point
65
to its corresponding character, which is 'A'.
Handling Invalid Unicode Code Points
Understand that valid Unicode code points range from
0
to1,114,111
(0x10FFFF
in hexadecimal).Attempt to convert an integer outside this range and handle the
ValueError
.pythontry: invalid_code = 1114112 print(chr(invalid_code)) except ValueError as e: print(f"Error: {e}")
If you try to convert
1114112
, which is beyond the valid range, Python raises aValueError
. This code handles the error and prints the error message.
Advanced Applications of chr()
Generating Alphabet Sequences
Use a loop to iterate through a range of Unicode code points corresponding to the alphabet.
Convert each code point to a character using
chr()
and store the results in a list.pythonstart = ord('a') end = ord('z') alphabet = [chr(i) for i in range(start, end + 1)] print("Alphabet:", ''.join(alphabet))
This loop generates a list of characters from
a
toz
using their corresponding Unicode code points. It uses list comprehension for concise and clean code.
Create Custom Character Mappings
Map specific integers to characters for custom encodings or decodings.
Use a dictionary to maintain a clear and modifiable mapping.
pythoncustom_mapping = {1: chr(97), 2: chr(98), 3: chr(99)} print("Custom Mapping:", custom_mapping)
This example creates a custom mapping where integers
1
,2
, and3
are mapped to Unicode characters 'a', 'b', and 'c', respectively.
Conclusion
The chr()
function in Python is a straightforward yet powerful tool for converting Unicode code points into characters. Its simplicity allows for effortless integration into text processing tasks, enhancing the functionality of Python scripts dealing with character data. By mastering the chr()
function, you enhance your capability to work with text data, create custom mappings, and implement advanced character manipulation techniques. Try incorporating the techniques discussed here to handle various character encoding scenarios in your projects effectively.
No comments yet.