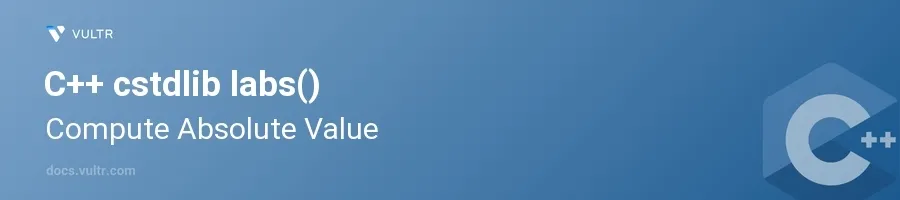
Introduction
The labs()
function in C++ is a C++ Standard Library function available through the <cstdlib>
header. This function computes the absolute value of a long integer and can be accessed using either the global scope (::labs
) or the std
namespace (std::labs
). As the function provides absolute values, it is crucial in various programming scenarios, from scientific computations to everyday mathematical operations.
In this article, you will learn how to use the labs()
function to compute absolute values in your C++ programs. Explore how this function handles different types of input values and see practical examples that demonstrate its usefulness in real-world programming situations.
Understanding the C++ labs() Function
Basic Usage of C++ labs() Function
Include the
<cstdlib>
header in your C++ program sincelabs()
is defined there.Call the
labs()
function with a long integer as its argument.cpp#include <cstdlib> #include <iostream> int main() { long int number = -1234567890L; long int absValue = labs(number); std::cout << "The absolute value is: " << absValue << std::endl; return 0; }
In this example,
labs()
computes the absolute value of-1234567890L
, which results in1234567890L
. The function efficiently turns a negative value into its positive counterpart.
Handling Different Value Types
Check if passing different types, like
int
, tolabs()
causes any issues.Understand that implicit conversions from
int
tolong int
may occur.cpp#include <cstdlib> #include <iostream> int main() { int smallNum = -123; long int absSmallNum = labs(smallNum); std::cout << "The absolute value of int value is: " << absSmallNum << std::endl; return 0; }
Here, an
int
variablesmallNum
implicitly converts tolong int
when passed tolabs()
, and the absolute value is correctly computed.
Edge Cases to Consider With C++ labs() Function
Consider what happens if the smallest possible
long int
value is passed tolabs()
.Understand that there might be an issue due to the limit of
long int
.cpp#include <cstdlib> #include <iostream> #include <limits> int main() { long int minLong = std::numeric_limits<long int>::min(); long int absMinLong = labs(minLong); std::cout << "Absolute value of min long int: " << absMinLong << std::endl; return 0; }
This example explores the behavior when the absolute function
labs()
is applied to the smallest possiblelong int
. Due to the symmetric range of negative and positive numbers, it might not return a correct positive equivalent, illustrating the need for careful handling of edge cases.
Conclusion
The labs()
function in C++ is an invaluable tool for computing the absolute values of long integers. By integrating this function into your programs, you streamline code related to mathematical computations and increase both readability and reliability. Ensure to handle edge cases and understand implicit type conversions to make the most out of this function. With these examples and insights, leverage labs()
effectively in various scenarios involving absolute value computations.
No comments yet.