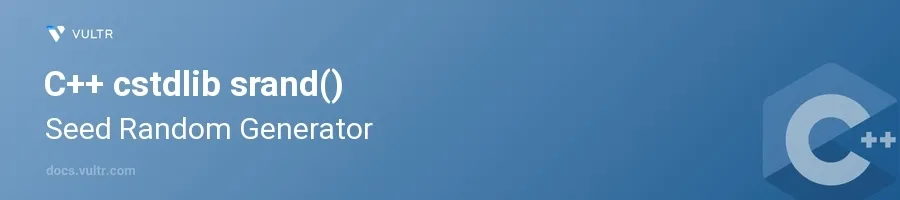
Introduction
The srand()
function in C++ is part of the <cstdlib>
library and plays a crucial role in generating pseudo-random numbers. It sets the starting point for producing a series of random integers by seeding the random number generator. This process is essential for ensuring that the sequence of random numbers varies with each execution of the program.
In this article, you will learn how to effectively use srand()
to seed the random number generator in C++. Explore how seeding affects the generation of random numbers and see practical examples demonstrating the use of srand()
in various scenarios.
Understanding srand()
Basics of srand()
- Recognize that
srand()
requires an argument in the form of an unsigned integer to seed the random number generator. - Understand that without seeding, the
rand()
function might produce the same sequence of numbers every time the program runs.
Seed the Random Number Generator
Include the necessary headers. For
srand()
, include<cstdlib>
, and for displaying results, include<iostream>
.cpp#include <cstdlib> #include <iostream>
Use the
srand()
function with a dynamic seed value, often derived from the current time, to ensure a different sequence of random numbers in each program run.cpp#include <ctime> // Include ctime to access the current time int main() { std::srand(std::time(0)); // Seed the random generator with current time int random_value = std::rand(); // Generate a random number std::cout << "Random Number: " << random_value << '\n'; return 0; }
After seeding the random generator with the current time using
std::time(0)
, the program generates a pseudo-random number. Each execution should now yield a different value.
Effects of Constant Seeding
Realize that using a constant seed results in the same sequence of random numbers across different runs of the program.
cppint main() { std::srand(42); // Constant seed value for (int i = 0; i < 5; ++i) { std::cout << "Random Number: " << std::rand() << '\n'; } return 0; }
In this example, the same sequence is created each time you run the program because the seed value does not change.
Practical Applications of srand()
Gaming: Seed for Fair Play
Use dynamic seeding in gaming applications to ensure different outcomes, which is vital for fairness and unpredictability:
cppstd::srand((unsigned) std::time(0));
Scientific Simulations
In simulations, control seed for reproducible results when needed, or use dynamic seeds for diverse outcomes.
cppstd::srand(42); // For reproducible results
Swap to dynamic seeds when examining variability across simulations:
cppstd::srand((unsigned) std::time(0));
Conclusion
The srand()
function in C++ is critical for influencing the behavior of the rand()
function by seeding the random number generator. Proper seeding ensures that truly random sequences are generated, which is essential in various applications such as gaming, simulations, and any functionality requiring randomized behavior. By mastering the use of srand()
, you enhance the robustness and unpredictability of your C++ programs, making them more dynamic and secure.
No comments yet.