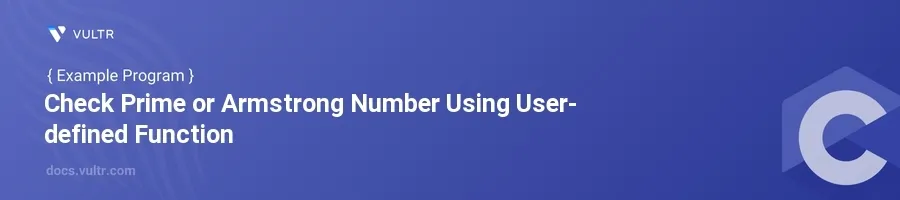
Introduction
In C programming, creating functions for specific tasks like checking for prime numbers or Armstrong numbers can make your code more organized and reusable. A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. An Armstrong number (also known as a narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits.
In this article, you will learn how to implement user-defined functions in C to check for prime numbers and Armstrong numbers. Practical examples will guide you through the process of setting up these functions and utilizing them effectively in your programs.
Creating the Prime Number Checker Function
Define the Prime Number Function
Define a function that accepts an integer and returns 1 if the number is prime and 0 if it is not. This function will iterate up to the square root of the number to check for factors.
c#include <stdio.h> #include <math.h> int isPrime(int num) { if (num <= 1) return 0; for (int i = 2; i <= sqrt(num); i++) { if (num % i == 0) return 0; } return 1; }
Here, the
isPrime
function checks each number from 2 to the square root ofnum
. If any number dividesnum
without a remainder, the function returns0
, indicating the number is not prime. If no divisors are found, it returns1
.
Using the Prime Number Function
Utilize the created function within a main program to check if a number is prime.
cint main() { int number; printf("Enter a number to check if it is prime: "); scanf("%d", &number); if (isPrime(number)) printf("%d is a prime number.\n", number); else printf("%d is not a prime number.\n", number); return 0; }
In this implementation, the user inputs a number, which is then passed to the
isPrime
function. The output informs whether the number is prime.
Creating the Armstrong Number Checker Function
Define the Armstrong Number Function
Define a function that identifies whether a number is an Armstrong number. This involves determining the number of digits, then checking if the sum of each digit raised to that power equals the original number.
cint isArmstrong(int num) { int original = num, sum = 0, digits = 0, temp; while (num != 0) { digits++; num /= 10; } num = original; while (num != 0) { temp = num % 10; sum += pow(temp, digits); num /= 10; } return (original == sum); }
This function first counts the digits in
num
. It then sums the cubes of each digit and compares this sum to the original number. If they are the same, it returns1
.
Using the Armstrong Number Function
Add functionality in your main program to check if a number is an Armstrong number.
cint main() { int number; printf("Enter a number to check if it is an Armstrong number: "); scanf("%d", &number); if (isArmstrong(number)) printf("%d is an Armstrong number.\n", number); else printf("%d is not an Armstrong number.\n", number); return 0; }
Similar to the prime check, this snippet allows the user to input a number and utilizes the
isArmstrong
function to check if it's an Armstrong number.
Conclusion
Mastering user-defined functions in C for specific checks like determining prime or Armstrong numbers not only enhances program cleanliness but also improves its modularity and reusability. By incorporating these examples into C programs, you enhance both the functionality and educational value of your code, making complex checks more manageable and intuitive. Use these functions to refine your approach to solving problems in C, streamlining your codebase effectively.
No comments yet.