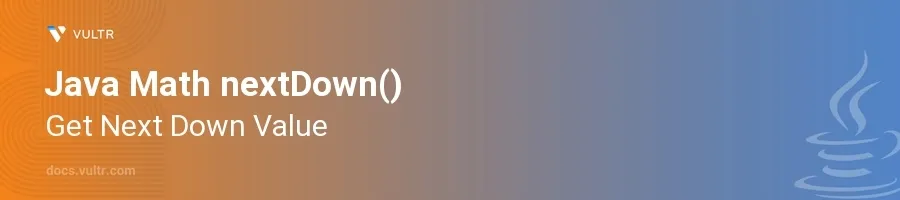
Introduction
The nextDown()
method in Java's Math class is particularly useful for finding the next smaller floating-point value toward negative infinity, from the given number. This function helps in situations where precise decrementing of values is crucial, such as graphics positioning, scientific calculations, or when working with multiple floating-point comparisons where precision is paramount.
In this article, you will learn how to effectively utilize the nextDown()
method in Java. You'll discover how this method can be applied to both single and double precision numbers, along with illustrative examples to demonstrate its practical usage in different scenarios.
Understanding nextDown() Method
Basic Usage of nextDown() for Double Values
Begin by initializing a double variable.
Apply the
nextDown()
function to decrement the value.javadouble initialValue = 1.0; double nextDownValue = Math.nextDown(initialValue); System.out.println("Next down value of 1.0 is: " + nextDownValue);
This snippet shows how to obtain the next smaller double-precision value from
1.0
. The method calculates the smallest decrement possible in floating-point representation.
Exploring nextDown() with Positive and Negative Values
Set both positive and negative double values.
Use
nextDown()
to observe how this function operates in both directions.javadouble positiveValue = 0.01; double nextDownPositive = Math.nextDown(positiveValue); double negativeValue = -0.01; double nextDownNegative = Math.nextDown(negativeValue); System.out.println("Next down value of 0.01 is: " + nextDownPositive); System.out.println("Next down value of -0.01 is: " + nextDownNegative);
This code evaluates
nextDown()
results for small positive and negative double values, showing that the function effectively moves values toward negative infinity.
Precision Considerations in Scientific Calculations
Demonstrate precision impact using
nextDown()
with a very small positive number.Print results to showcase the high-precision calculation.
javadouble verySmallValue = 1e-10; // 1 * 10^-10 double result = Math.nextDown(verySmallValue); System.out.println("Next down from very small value: " + result);
The output will reveal the tiny decrement done on a very small number. This precision is significant in fields requiring high accuracy, like physics simulations.
Using nextDown() with Float Values
Basic Application for Float Types
Initialize a float variable.
Use
nextDown()
specifically for the float type.javafloat initialFloat = 0.5f; float nextDownFloat = Math.nextDown(initialFloat); System.out.println("Next down value of 0.5f is: " + nextDownFloat);
Unlike double precision, this example works with a single precision float, adjusting the least significant bit of its representation.
Handling Extremes in Float Calculations
Evaluate the behavior of
nextDown()
with extreme float values, such asFloat.MIN_VALUE
.javafloat minFloat = Float.MIN_VALUE; float extremeNextDown = Math.nextDown(minFloat); System.out.println("Next down value of Float.MIN_VALUE is: " + extremeNextDown);
This demonstrates that
nextDown()
could even result in values that underflow, leading to effects like negative zeros, which are important in certain computing contexts.
Conclusion
The nextDown()
function in Java provides an essential capability to decrement floating-point values precisely, ensuring the minimal possible decrease toward negative infinity. By leveraging this function, you maintain high accuracy in applications that demand ultraprecision, from graphical adjustments on the pixel level to meticulous scientific calculations. Implement these techniques to enhance reliability and precision in your Java programs that involve detailed floating-point arithmetic.
No comments yet.