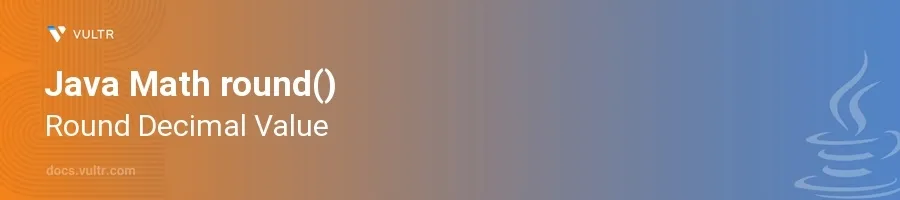
Introduction
The Math.round()
method in Java is a straightforward yet powerful utility for rounding floating-point numbers to the nearest integer. It is part of the Java Math library, which provides numerous functions for performing mathematical operations, making it indispensable for developers dealing with calculations. Understanding how to use Math.round()
correctly can help in ensuring numerical data adheres to expected precision and formats.
In this article, you will learn how to utilize the Math.round()
method to round various types of decimal values effectively. Discover the nuances between rounding double and float datatypes and explore practical examples to solidify your understanding of this method.
Rounding Float Values
Basic Rounding of Floats
Declare a float variable.
Apply the
Math.round()
method.javafloat value = 123.456f; int rounded = Math.round(value); System.out.println("Rounded value: " + rounded);
This code snippet rounds the float value
123.456
to123
becauseMath.round()
rounds to the nearest integer.
Rounding Negative Floats
Understand that the rounding behavior is consistent even with negative numbers.
Round a negative float value.
javafloat negativeValue = -123.456f; int roundedNegative = Math.round(negativeValue); System.out.println("Rounded negative value: " + roundedNegative);
Here,
Math.round()
rounds the negative float-123.456
to-123
. The method rounds towards the nearest whole number, adhering to standard rounding rules.
Rounding Double Values
Basic Rounding of Doubles
Declare a double variable.
Use
Math.round()
to round it.javadouble doubleValue = 987.654321; long roundedDouble = Math.round(doubleValue); System.out.println("Rounded double: " + roundedDouble);
In this example, the double
987.654321
is rounded to988
. When usingMath.round()
on a double, the result is of typelong
.
Handling Very Large Doubles
Consider the limitations of the data type when rounding large double values.
Round a very large double value and observe the result.
javadouble largeValue = 9e11; // 900 billion long roundedLargeDouble = Math.round(largeValue); System.out.println("Rounded very large double: " + roundedLargeDouble);
This code illustrates rounding a large double value, 900 billion (
9e11
), to a long integer. The result remains precise due to the capabilities of thelong
type in Java, which can handle such large numbers.
Conclusion
The Math.round()
method in Java is essential for rounding floating-point numbers to their nearest integer values. This function operates effectively across both float and double data types, ensuring accuracy whether dealing with positive or negative numbers, or even very large values. Implementing Math.round()
helps achieve numerical precision and formatting in applications, enhancing data presentation and processing accuracy. By exploring the examples provided, you can confidently apply this method in various contexts of your Java projects.
No comments yet.