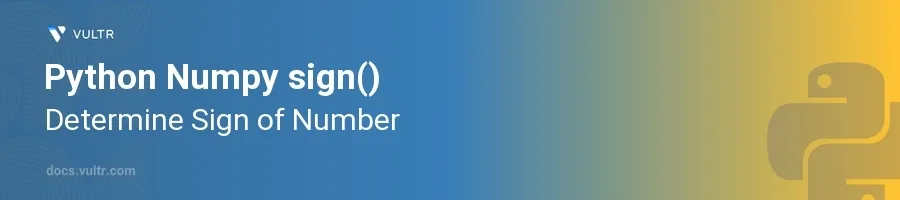
Introduction
The numpy.sign()
function is a versatile tool in the NumPy library used to determine the sign of a number, which includes negative, positive, or zero categories. This function is particularly useful in data processing and mathematical computations where the behavior of different values needs to be identified or separated based on their signs.
In this article, you will learn how to use the numpy.sign()
function effectively in Python to handle various data types including single numbers, lists, and numpy arrays. Additionally, you'll explore the function's behavior when dealing with complex numbers.
Using numpy.sign() with Different Data Types
Determining the Sign of a Single Number
Import the NumPy library.
Define a single number.
Apply the
numpy.sign()
function to identify its sign.pythonimport numpy as np num = -8 sign_of_num = np.sign(num) print(sign_of_num)
In this example, since the number is negative,
numpy.sign()
returns-1
.
Working with Lists of Numbers
Use a list of integers and pass it directly to
numpy.sign()
using numpy array conversion.Evaluate the list to see the sign for each element.
pythonimport numpy as np list_nums = [-1, 0, 1, 5, -5] signs = np.sign(list_nums) print(signs)
This code converts the list of numbers into a Numpy array and determines the sign for each number, returning an array of signs.
Handling Numpy Arrays
Create a numpy array from a sequence of numbers.
Apply the
numpy.sign()
function on the entire array.pythonimport numpy as np array_nums = np.array([-10, 0, 10, 20, -3]) sign_array = np.sign(array_nums) print(sign_array)
Here,
numpy.sign()
is used on a numpy array. It processes each element and outputs an array showing the sign of each element.
Dealing with Complex Numbers
Understand that
numpy.sign()
returns 1 for any complex number where the real part is not zero.Implement this with a complex number example.
pythonimport numpy as np complex_num = 3 + 4j sign_complex = np.sign(complex_num) print(sign_complex)
The function evaluates the complex number and returns a normalized value of
1 + 0j
, indicating the non-zero real part.
Conclusion
The numpy.sign()
function in Python provides a straightforward and efficient method for determining the sign of numerical data, whether they be in single-value formats, lists, arrays, or even complex numbers. Its broad applicability makes it an essential function in the NumPy library for both simple and complex mathematical tasks. Gain insights into the behavior of data and simplify conditional logic in data processing by leveraging this function to its full potential.
No comments yet.