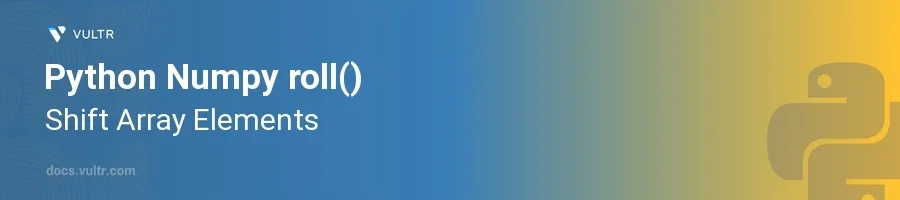
Introduction
The numpy.roll()
function in Python provides a straightforward way to shift the elements of an array, either along a specified axis or for the array as a whole. This technique is particularly useful in various mathematical computations and data manipulation tasks, where adjusting the element positions can derive new insights or desired data structures.
In this article, you will learn how to effectively use the numpy.roll()
function to shift array elements. Explore practical examples that demonstrate shifting elements in one-dimensional and multi-dimensional arrays, including how to control the direction and magnitude of shifts.
Using numpy roll() with One-dimensional Arrays
Basic Element Shifting
Import the numpy library.
Define a one-dimensional numpy array.
Apply
numpy.roll()
to shift the array elements.pythonimport numpy as np arr = np.array([1, 2, 3, 4, 5]) rolled_arr = np.roll(arr, 2) print(rolled_arr)
This code shifts each element in the array
arr
two positions to the right. The output will be[4, 5, 1, 2, 3]
.
Negative Shifts
Use a negative shift value to move elements to the left.
pythonnegative_rolled_arr = np.roll(arr, -2) print(negative_rolled_arr)
This configuration moves each element in the array
arr
two positions to the left, resulting in[3, 4, 5, 1, 2]
.
Using numpy roll() with Multi-dimensional Arrays
Shifting Elements Across Columns
Create a two-dimensional numpy array.
Perform a column-wise shift using the
axis
argument.pythonmatrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) rolled_matrix = np.roll(matrix, shift=1, axis=1) print(rolled_matrix)
This code shifts elements in each row one position to the right across columns. The output is
[[3, 1, 2], [6, 4, 5], [9, 7, 8]]
.
Shifting Elements Across Rows
Shift elements in a vertical manner within a 2D array.
pythonrolled_matrix_rows = np.roll(matrix, shift=1, axis=0) print(rolled_matrix_rows)
Here, elements are shifted down by one position along the rows. The modified array
[7, 8, 9]
moves to the top, resulting in[[7, 8, 9], [1, 2, 3], [4, 5, 6]]
.
Conclusion
The numpy.roll()
function is an effective tool for shifting array elements, offering flexibility to handle both simple and complex data structures. Whether adjusting elements in a single-dimensional list or performing intricate shifts in multi-dimensional arrays, numpy.roll()
supports a wide range of applications. Utilize this function to manipulate data structures effortlessly, ensuring your numerical computations and data analyses remain robust and adaptable.
No comments yet.