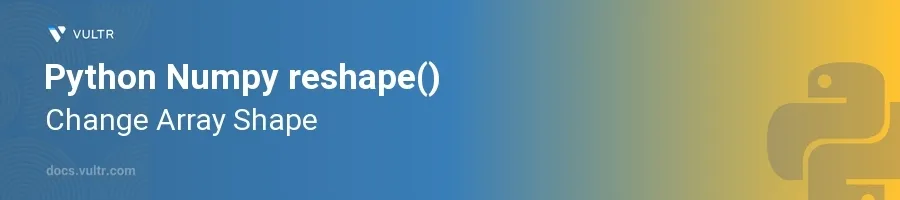
Introduction
The reshape()
function in NumPy is a fundamental tool for transforming the shape of arrays without changing their data. This capability is crucial in data manipulation and preprocessing, especially in tasks involving image processing, machine learning, and scientific computing where the organization of data can greatly impact the performance and ease of analysis.
In this article, you will learn how to utilize the NumPy reshape()
method to alter the dimensions of arrays effectively. Explore different scenarios including reshaping one-dimensional arrays to two-dimensional grids, changing the size of images for machine learning models, and dealing with higher-dimensional data.
Understanding reshape()
Basic Reshaping from 1D to 2D
Start by importing NumPy.
Create a one-dimensional array.
Reshape it into a two-dimensional array using
reshape()
.pythonimport numpy as np one_d_array = np.arange(10) # Creates an array [0, 1, 2, ..., 9] two_d_array = one_d_array.reshape((2, 5)) print(two_d_array)
This snippet reshapes a one-dimensional array into a two-dimensional array with 2 rows and 5 columns. The resultant shape must accommodate all elements from the original array.
Changing Shape Dynamically
Use
-1
inreshape()
to automatically calculate the dimension size.Define an array and reshape it by specifying one dimension explicitly and the other as
-1
.pythondata = np.arange(24) reshaped_data = data.reshape((4, -1)) print(reshaped_data)
In this example, NumPy calculates the unnamed dimension (
-1
) such that the total size remains constant. The array changes into a 4x6 matrix.
Reshaping Three-Dimensional Data
Understand how to manipulate more complex structures.
Reshape an array into three dimensions.
pythonthree_d_data = np.arange(24) reshaped_to_3d = three_d_data.reshape((2, 3, 4)) print(reshaped_to_3d)
Here, the array is reshaped into a three-dimensional array of dimensions 2x3x4. Such transformations are useful in applications like computer graphics, where volumetric representations are needed.
Common Errors and Precautions
Ensuring Compatibility of Dimensions
Ensure the total number of elements matches the new shape.
Check the reshaped array size to see if it fits the original.
pythontry: np.arange(10).reshape((3, 4)) except ValueError as e: print("Error:", e)
This code throws a
ValueError
because reshaping a 10-element array to a 3x4 matrix (which requires 12 elements) is not possible.
Benefits of Not Copying Data
Note that
reshape()
usually returns a view, not a copy.Understand this behavior helps in designing memory-efficient programs.
pythonoriginal_array = np.arange(6) reshaped_array = original_array.reshape((2, 3)) reshaped_array[0, 0] = 999 print(original_array)
Changes to the reshaped array reflect in the original array, indicating both share the same data memory.
Conclusion
Using the reshape()
function in NumPy effectively changes the dimensions of arrays without altering the underlying data, facilitating seamless data manipulation for diverse computational tasks. Whether working with simple 2D transformations or complex 3D models, mastering reshape()
enhances your ability to handle array data proficiently. Adopt these techniques to ensure you can adapt data shapes to fit the requirements of various algorithms and processing frameworks, improving the robustness and flexibility of your code.
No comments yet.