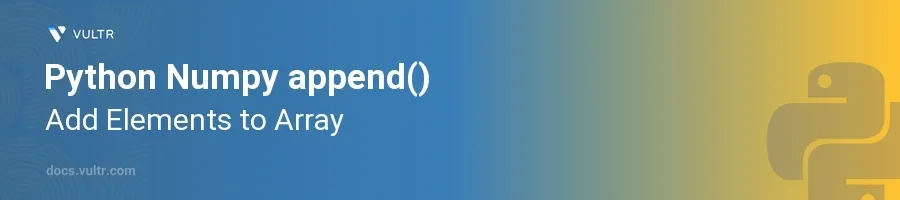
Introduction
The numpy.append()
function in Python is an essential tool for data manipulation in scientific computing. It enables the addition of values to the end of a NumPy array, which is a frequent requirement when processing or transforming data iteratively. Whether you're working on data analysis, machine learning algorithms, or regular data operations, append()
becomes critical in handling and modifying array structures efficiently.
In this article, you will learn how to use the numpy.append()
function to append elements to a numpy array. You will gain insights into appending elements to both one-dimensional and multi-dimensional arrays and understand the implications on array shape and efficiency.
Appending to One-dimensional Arrays
Initialize the Array
Begin with a simple one-dimensional numpy array append operation.
Plan to append elements to this array.
pythonimport numpy as np initial_array = np.array([1, 2, 3])
Append Elements
Use
numpy.append()
to add a single element or multiple elements.pythonupdated_array = np.append(initial_array, [4, 5]) print(updated_array)
This snippet demonstrates how to append to a numpy array by taking the
initial_array
and appending the elements[4, 5]
to it. The result is a new array[1, 2, 3, 4, 5]
. If you're looking to add an element to a numpy array,np.append()
is the way to do it.
Appending to Multi-dimensional Arrays
Understanding Array Dimensions
- Recognize that
np.append()
does not modify the array in-place but returns a new array. If you're experiencing issues wherenp.append
is not working, ensure the operation aligns with the expected dimensions. - Consider the dimensions when appending to ensure the expected array shape.
Append Elements to a Two-dimensional Array
Start with a two-dimensional array.
Append another array to it, keeping the dimensions in consideration.
pythontwo_dim_array = np.array([[1, 2], [3, 4]]) array_to_append = np.array([[5, 6]]) new_two_dim_array = np.append(two_dim_array, array_to_append, axis=0) print(new_two_dim_array)
Here, the function performs
numpy append to array
by addingarray_to_append
along the first axis (rows). The resulting array will have an increased number of rows but retain the same number of columns.
Handling Inconsistent Shapes
Ensure the array shapes are compatible when applying a
numpy append element to array
operation along a specific axis.Use
axis=None
if merging arrays into a single dimension is acceptable.pythonresult_array = np.append(two_dim_array.flatten(), array_to_append.flatten()) print(result_array)
Flattening the arrays before performing
numpy array append
ensures that incompatible shapes do not cause issues. This method helps append an array in Python while avoiding shape mismatches.
Handling Performance Considerations When Using the numpy.append()
function in Python
Understanding Performance Issues
- Using
numpy append
repeatedly creates a new array each time, which can slow down performance. - If the np.append function is not working as expected, excessive memory usage may be the cause.
- Instead of repeatedly using
python np append
, consider more efficient ways to append to numpy array.
Optimize numpy append
Usage
Instead of calling numpy append array multiple times, collect elements first and then append.
If you need to add element to numpy array frequently, preallocate space when possible.
pythonimport numpy as np temp_array = np.array([1, 2, 3]) new_data = [4, 5, 6] result_array = np.append(temp_array, new_data) print(result_array)
Here,
np.append python
efficiently combinestemp_array
andnew_data
in a single operation, reducing repeated memory reallocation.
Preallocating Arrays for Efficiency
If you need to append numpy array multiple times, preallocating space avoids unnecessary copying.
When working with large datasets, modifying an existing
np array append
is more efficient.pythonpreallocated_array = np.empty(6) # Preallocate an array with 6 elements preallocated_array[:3] = [1, 2, 3] preallocated_array[3:] = [4, 5, 6] print(preallocated_array)
Preallocating space helps avoid excessive calls to
numpy append element to array
, making operations more efficient.
Using Lists Before Appending to a Array
If you don't know the final array size, use lists before converting to
numpy array append
.Lists handle dynamic resizing better than calling
append numpy array
repeatedly.pythontemp_list = [1, 2, 3] temp_list.extend([4, 5, 6]) # Use Python lists to accumulate elements final_array = np.array(temp_list) # Convert to NumPy array at the end print(final_array)
Here,
python append to numpy array
is done efficiently by appending elements to a list first, then converting it to a NumPy array in one step.
By applying these strategies, you can use numpy append
effectively while avoiding unnecessary slowdowns in large datasets.
Conclusion
The numpy.append()
function is a versatile tool for expanding numpy arrays across various dimensions and is pivotal in data manipulation tasks. Remember, it generates a new array each time it is called, which may impact performance in large-scale data operations. Apply these methods to integrate additional data into existing arrays or to aggregate results from iterative processes. By mastering numpy.append()
, you enhance your ability to efficiently manipulate large datasets within Python.
No comments yet.