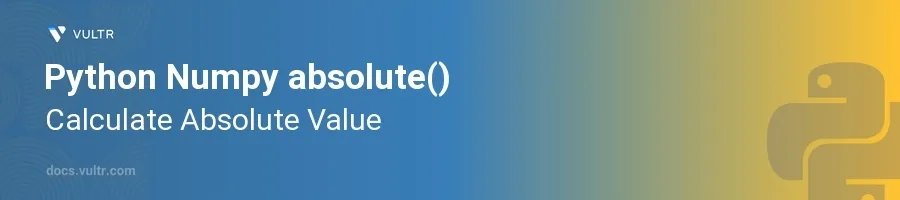
Introduction
The numpy.absolute()
function in Python, part of the NumPy library, is designed to calculate the absolute values of the elements in arrays. This function proves invaluable when dealing with numeric data that requires non-negative values for analysis, such as distances, magnitudes, and physical quantities.
In this article, you will learn how to apply the numpy.absolute()
function across different data types and structures in Python, enhancing your ability to handle and manipulate numerical data effectively in arrays and matrices.
Getting Started with numpy.absolute()
Applying the Function to a Single Array
Import the NumPy library.
Create a numpy array with both positive and negative numbers.
Calculate the absolute values using the
numpy.absolute()
function.pythonimport numpy as np data = np.array([-1, -2, 3, -4]) abs_values = np.absolute(data) print(abs_values)
This code converts all negative numbers in the
data
array to their positive equivalents, ensuring all values are non-negative.
Dealing with Multi-dimensional Arrays
Recognize that
numpy.absolute()
can handle arrays of any dimension.Construct a multi-dimensional array with various integer values.
Apply the absolute function to see the effect across dimensions.
pythonmulti_dim_data = np.array([[-3, -7, 2], [9, -8, -4]]) abs_multi_dim_values = np.absolute(multi_dim_data) print(abs_multi_dim_values)
This example demonstrates how the absolute values are calculated element by element, irrespective of the array's dimensionality.
Using numpy.absolute() with Complex Numbers
Working with Complex Number Arrays
Understand that absolute value of complex numbers refers to their magnitude.
Prepare an array of complex numbers.
Execute the absolute function to determine the magnitudes.
pythoncomplex_data = np.array([3+4j, -2-1j, 0+1j]) abs_complex_values = np.absolute(complex_data) print(abs_complex_values)
The output provides the magnitudes of the complex numbers in the array. For instance,
3+4j
has a magnitude calculated assqrt(3^2 + 4^2)
.
Conclusion
The numpy.absolute()
function is a versatile tool for computing the absolute values of elements within numpy arrays, including handling complex data types. Whether working with simple or complex datasets, single or multi-dimensional arrays, this function ensures that the data handling processes in Python remain robust and efficient. Leverage this functionality to streamline tasks in scientific computing and data analysis where absolute values are critical.
No comments yet.