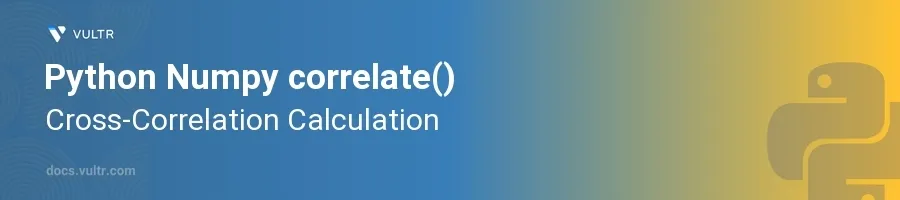
Introduction
The numpy.correlate()
function is a key tool in signal processing, used to compute the cross-correlation of two 1-dimensional sequences. This method proves especially beneficial in various fields such as economics, physics, and engineering, where it helps in identifying the relationship between two time series datasets. It measures how much one series is similar to another by sliding one sequence over another and computing sums of products.
In this article, you will learn how to leverage the numpy.correlate()
method effectively. Explore how to perform basic operations, understand the interpretations of its results, and how to apply it to real-world data analysis and signal processing.
Basic Usage of numpy.correlate()
Calculating Cross-Correlation Between Two Sequences
Import the numpy library.
Declare two numeric arrays that represent the sequences.
Apply the
numpy.correlate()
function with appropriate parameters.pythonimport numpy as np a = np.array([1, 2, 3]) b = np.array([0, 1, 0.5]) cross_corr = np.correlate(a, b, 'full') print(cross_corr)
In this code, two arrays
a
andb
are defined. The'full'
mode is used innp.correlate()
, which provides the complete cross-correlation sequence. The result will show how each element ofa
correlates with each element ofb
across different time shifts.
Understanding Output Modes
Know the different modes:
full
,valid
, andsame
.Use each mode to see how the output changes.
pythonfull_mode = np.correlate(a, b, 'full') valid_mode = np.correlate(a, b, 'valid') same_mode = np.correlate(a, b, 'same') print("Full mode:", full_mode) print("Valid mode:", valid_mode) print("Same mode:", same_mode)
The output will change based on the mode specified.
full
gives the correlation at each shift,valid
gives the correlation where sequences fully overlap, andsame
gives the correlation where the output size is the same as the largest input.
Real-World Applications
Signal Time Delay Estimation
Create two signals where one is a delayed version of the other.
Compute the cross-correlation between these signals.
Find the lag at which the correlation is highest to estimate time delay.
pythonx = np.arange(0, 10) y = np.roll(x, 2) # Delay signal 'x' by 2 correlation = np.correlate(x, y, 'full') estimated_delay = np.argmax(correlation) - (len(x) - 1) print(f"Estimated Time Delay: {estimated_delay} units")
In this example,
y
is a delayed version ofx
by 2 units. Thenp.argmax()
function finds the index of the maximum value in the correlation array, from which the estimated time delay is extracted by adjusting for the length ofx
.
Conclusion
The numpy.correlate()
function is immensely powerful for analyzing the relationship between two time series, particularly in fields like signal processing and time series analysis. Use this function to detect similarities or calculate delays between signals, enhancing insights from data. Mastery over settings such as mode parameters equips you with greater control over the correlation process, allowing for tailored analysis that fits specific requirements of any task.
No comments yet.