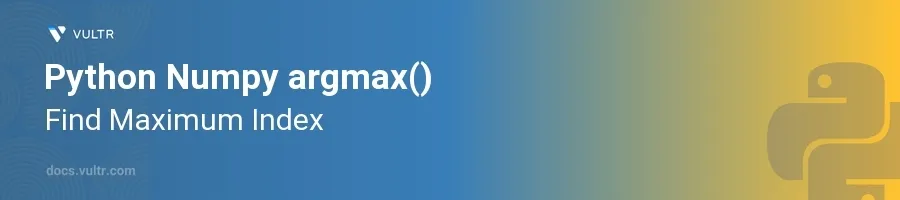
Introduction
The numpy.argmax()
function in Python is a powerful tool within the NumPy library, designed to return the indices of the maximum values along the specified axis. Navigating arrays and matrices to find the index of the maximum value in an array is a routine task in data analysis, machine learning preprocessing, and general programming, where decisions based on the highest scores are required.
In this article, you will learn how to effectively harness the numpy.argmax()
function. Explore its capabilities in handling one-dimensional arrays, multi-dimensional arrays, and complex scenarios where comparison of elements is essential. Understand how to customize its behavior by specifying axes and see practical examples that demonstrate its use in various contexts.
Understanding numpy.argmax() Basics
Applying argmax() to One-dimensional Arrays
Import the NumPy library.
Initialize a one-dimensional array.
Use
np.argmax()
to find the index of the max value in a numpy array.pythonimport numpy as np array1d = np.array([10, 20, 90, 40, 50]) max_index = np.argmax(array1d) print("Index of maximum element:", max_index)
In this example,
np.argmax()
identifies the maximum value90
in the array and returns its index, which is2
. This demonstrates how to get the index of the max value in a NumPy array.
Utilizing argmax() with Multi-dimensional Arrays
Consider a two-dimensional array.
Apply
np.argmax()
without specifying an axis to find the overall maximum index.Use the
axis
parameter to get the index of the max value in a 2D NumPy array along a specified axis.pythonarray2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) overall_max_index = np.argmax(array2d) column_max_indices = np.argmax(array2d, axis=0) row_max_indices = np.argmax(array2d, axis=1) print("Overall maximum index:", overall_max_index) print("Max indices per column:", column_max_indices) print("Max indices per row:", row_max_indices)
When
np.argmax()
is applied to a 2D array without an axis, it returns the index of the max value as if the array were flattened. Specifyingaxis=0
finds the indices of maximum values in columns, whereasaxis=1
gives the indices of max values per row.
Advanced Usage of argmax()
Using argmax() in Practical Scenarios
Imagine extracting the most frequent categories that appear in customer feedback categorization.
Analyze and identify indices where maximum values occur repeatedly across time-series data.
pythonarray_3d = np.array([[[1, 2, 3], [4, 5, 2]], [[1, 0, 3], [6, 5, 4]]]) time_series_max = np.argmax(array_3d, axis=2) print("Indices of maximum values in time-series data:", time_series_max)
This example traverses a three-dimensional array and finds the indices of the max values for each subarray in the last dimension, which could mimic real-life scenarios like time-stamped data in machine learning processes.
Conclusion
The numpy.argmax()
function is essential when you need to find the index of the maximum value in a NumPy array. Whether working with one-dimensional, two-dimensional, or multi-dimensional arrays, np.argmax()
provides a straightforward way to retrieve the index of max values efficiently.
Understanding how to find the max index in NumPy arrays enhances data analysis workflows, making them more streamlined and reliable. By specifying axis
in numpy.argmax()
, you can extract max indices in multi-dimensional arrays, such as finding the index of the max value in a 2D NumPy array or locating peak values in structured data.
No comments yet.