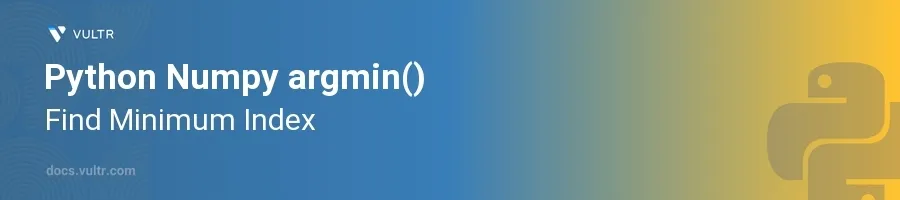
Introduction
The numpy.argmin()
function in Python is a tool for finding the index of the minimum value in an array. This function is integral to data analysis, machine learning preprocessing, and anywhere you need to identify the position of the smallest element quickly.
In this article, you will learn how to effectively utilize the numpy.argmin()
function in your Python code. Explore practical examples to see how this function operates on various data structures such as single-dimensional and multi-dimensional arrays.
Understanding numpy.argmin()
Basic Usage in Single-dimensional Arrays
Import the numpy library.
Create a single-dimensional numpy array.
Apply the
argmin()
function to find the index of the minimum value.pythonimport numpy as np array = np.array([10, 20, 5, 40]) min_index = np.argmin(array) print(min_index)
This code returns the index
2
because5
is the minimum value in the array, and it is located at the index2
.
Handling Multi-dimensional Arrays
Initialize a two-dimensional array.
Use
argmin()
to find the index of the minimum value across the entire array.Further, apply
argmin()
along a specific axis to find the indices of minimum values in that dimension.pythonmulti_array = np.array([[10, 2], [5, 20]]) overall_min = np.argmin(multi_array) column_min = np.argmin(multi_array, axis=0) row_min = np.argmin(multi_array, axis=1) print("Index of overall minimum:", overall_min) print("Indices of minimums in each column:", column_min) print("Indices of minimums in each row:", row_min)
In this snippet:
- The overall minimum value is
2
, andargmin()
returns1
as it is located at the second position if the array is flattened. - The column-wise minima are
5
and2
, with indices1
and0
respectively, in their columns. - The row-wise minima are
2
and5
, located at indices1
and0
in their respective rows.
- The overall minimum value is
Using argmin() with Conditioned Data
Establish the numpy array with varied data.
Apply a conditional operation to generate a boolean array.
Use
argmin()
to determine the firstTrue
position in the conditioned array.pythondata_array = np.array([10, -20, -5, 50, -60]) negative_cond = data_array < 0 first_negative_index = np.argmin(negative_cond == False) print("First index with a negative value:", first_negative_index)
Here,
argmin()
identifies the first index where the condition (data_array < 0
) becomesTrue
, thus pinpointing the first negative value in the data set.
Conclusion
The numpy.argmin()
function is a versatile tool for efficiently locating the index of minimum values within an array, providing valuable functionality across single and multi-dimensional data sets. Whether handling simple arrays or complex matrices, understanding how to leverage argmin()
enhances data analysis and preprocessing tasks effectively. Utilize these strategies to simplify searches for minimum values in your datasets, improving both performance and readability of your Python code.
No comments yet.