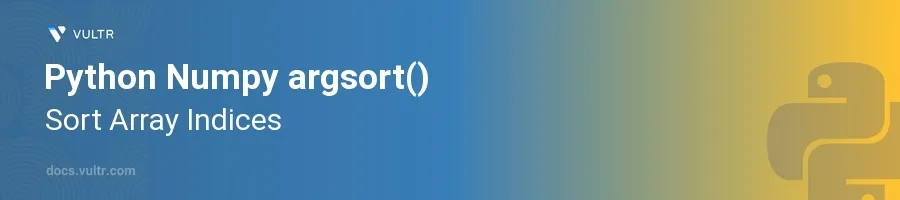
Introduction
The argsort()
function in NumPy is a powerful tool that returns the indices that would sort an array. This functionality is essential when you need to sort elements in one array and simultaneously arrange the corresponding elements in another array or when you want to understand the sorted order of elements without modifying the original array.
In this article, you will learn how to efficiently utilize the argsort()
function with various types of arrays in Python. Explore practical examples demonstrating the sorting of numeric data, managing arrays based on sorted indices, and applying these techniques to real-world scenarios.
Utilizing argsort() with Numeric Arrays
Sort Indices of a Simple Array
Initialize a NumPy array with arbitrary numeric values.
Use the
argsort()
function to get sorted indices.pythonimport numpy as np data = np.array([10, 1, 5, 3, 8, 6]) sorted_indices = data.argsort() print(sorted_indices)
This code snippet sorts the
data
array and returns the indices that sort the array. The output shows the indices that would position the elements in ascending order.
Reordering Arrays Using Sorted Indices
Retrieve sorted indices from a numeric array.
Use these indices to reorder the array.
pythonsorted_data = data[sorted_indices] print(sorted_data)
By using the indices obtained from
argsort()
,sorted_data
is now a sorted version of the originaldata
array, organizing the actual data elements in ascending order.
Applying argsort() in Complex Scenarios
Sorting Based on Secondary Criteria
Create a structured array with multiple fields.
Sort the array based on a primary field and then by a secondary field using indices from
argsort()
.pythonstructured_data = np.array([(3, 'b'), (1, 'a'), (2, 'c'), (2, 'a')], dtype=[('x', int), ('y', 'U1')]) primary_sort_indices = structured_data['x'].argsort() secondary_sort_subset = structured_data[primary_sort_indices]['y'].argsort(kind='stable') final_sorted = structured_data[primary_sort_indices][secondary_sort_subset] print(final_sorted)
This example first sorts the array based on the 'x' field, then sorts subsets of 'y' within the same 'x' values, resulting in both primary and secondary sorting criteria being applied effectively.
Use in Data Science for Feature Sorting
Assume a dataset where each row represents an entity and columns represent features.
Sort features of an entity based on their influence or value.
pythonfeatures = np.array([0.1, 0.6, 0.2, 0.8]) influential_features_indices = features.argsort()[::-1] print(influential_features_indices)
Sorting the indices in descending order allows identifying the most influential features, which is useful in tasks like feature selection in machine learning.
Conclusion
NumPy's argsort()
function is invaluable when you need to sort arrays by indices or apply complex sorting orders to multidimensional data. It simplifies tasks where the relationship between elements across different arrays or within arrays must be maintained after sorting. Implement the technique of indexing with sorted indices to enhance your data manipulation capabilities in Python, ensuring efficiency and simplicity in your data processing and analysis workflows.
No comments yet.