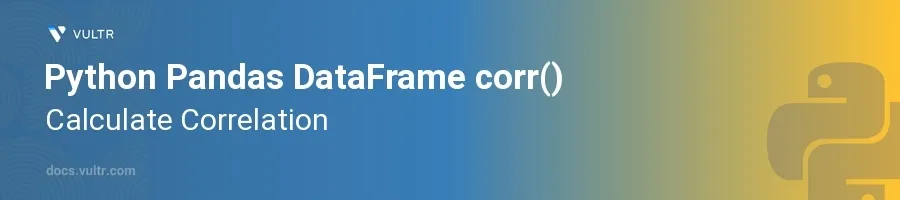
Introduction
In data analysis and statistics, understanding the correlation between different variables is crucial. The correlation measures how closely changes in one variable are associated with changes in a second variable. For this purpose, Python's Pandas library provides a powerful tool, the corr()
method, used extensively in financial analysis, social sciences, biology, and more to find relationships between data series.
In this article, you will learn how to utilize the corr()
method on a Pandas DataFrame to compute pairwise correlation of columns, excluding NA/null values. Discover different methods of correlation such as Pearson, Kendall, and Spearman, and see how to interpret and apply these techniques in your data science workflows.
Understanding Correlation Types
Before diving into code implementations, it's imperative to understand the types of correlation coefficients available and when to use them.
Pearson Correlation Coefficient
- Recognize that Pearson’s correlation measures the linear relationship between two continuous variables.
- Note that it assumes the normal distribution of the involved variables and is sensitive to outliers.
Kendall Tau Correlation Coefficient
- Accept that Kendall's tau is a non-parametric measure.
- Use it when the data does not necessarily follow a normal distribution and for ordinal data.
Spearman Rank Correlation Coefficient
- Understand that Spearman’s correlation assesses how well the relationship between two variables can be described using a monotonic function.
- Opt for this coefficient when your data is not normally distributed or is ordinal.
Applying corr() in Pandas
Basic Usage of corr()
Start by importing the Pandas library and creating a DataFrame.
Use the
corr()
method without parameters to default to the Pearson method.pythonimport pandas as pd data = { 'A': [1, 2, 3, 4, 5], 'B': [5, 4, 3, 2, 1], 'C': [2, 3, 4, 5, 6] } df = pd.DataFrame(data) correlation_matrix = df.corr() print(correlation_matrix)
This example constructs a DataFrame and calculates the Pearson correlation for each pair of columns. The result is a new DataFrame where each element is the correlation coefficient between two columns.
Using Different Methods
Modify the
corr()
method to use a different correlation coefficient such as Kendall or Spearman.Replace the default method by passing the appropriate method parameter.
pythonkendall_corr = df.corr(method='kendall') spearman_corr = df.corr(method='spearman') print("Kendall's correlation:") print(kendall_corr) print("Spearman's correlation:") print(spearman_corr)
Here, Kendall's tau and Spearman's rank coefficients are computed for the same DataFrame. The output will show different values, reflecting the different assumptions and calculations used by these methods.
Handling Missing Data
When dealing with real-world data, missing values are common. corr()
automatically excludes null or NA values from its calculations.
How to Verify and Handle Missing Data
Ensure your dataset is cleaned and preprocessed before applying
corr()
.Use DataFrame methods like
dropna()
orfillna()
to manage missing values, depending on your analysis requirements.pythondf_clean = df.dropna() # Removes rows with any missing values correlation_matrix_clean = df_clean.corr() print(correlation_matrix_clean)
In this snippet,
dropna()
is used to remove any rows with missing values before calculating correlations. This ensures that the correlation calculations are performed on complete cases only.
Conclusion
The corr()
method in Pandas is an essential function for statistical analysis, aiding in the discovery of relationships between pairs of continuous or ordinal variables. By understanding and properly applying the various correlation coefficients, you refine your data analysis, ensuring your conclusions are supported by the appropriate statistical tests. Apply the knowledge gained here to interpret complex datasets and derive meaningful insights about the relationships within your data. Whether you are handling financial datasets, social science surveys, or biological data, mastering corr()
enhances the robustness and depth of your analytical capabilities.
No comments yet.