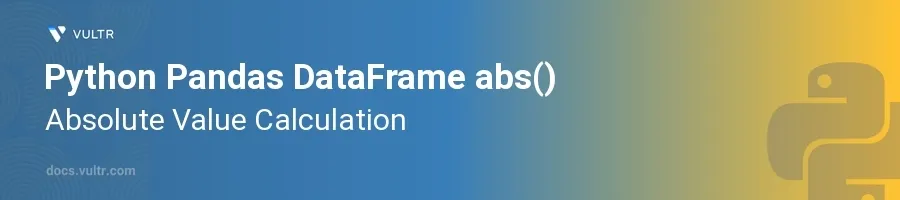
Introduction
The abs()
function in the context of Python's Pandas library is a valuable tool for handling numerical data, especially when manipulating DataFrames with numeric entries that include negative values. This function converts every entry to its absolute value, which can be particularly useful in data preprocessing, error analysis, and scenarios where the magnitude of values is more important than their sign.
In this article, you will learn how to effectively apply the abs()
method to DataFrames in various data manipulation scenarios. Explore how this function transforms a DataFrame by converting all the negative values to their positive counterparts and understand the impact of this operation on data analysis tasks.
Understanding the abs() Function
Basic Usage in DataFrames
Import the necessary library, create a DataFrame, and apply
abs()
.pythonimport pandas as pd df = pd.DataFrame({ 'A': [-1, 2, -3], 'B': [4, -5, 6] }) df_abs = df.abs() print(df_abs)
This simple example sets up a DataFrame with positive and negative numbers across multiple columns. Applying
abs()
converts all the entries to absolute values. The DataFramedf_abs
will display as:A B 0 1 4 1 2 5 2 3 6
Application in Data Analysis
Consider a more complex DataFrame.
Apply the
abs()
to explore data in terms of magnitude.pythondata = { 'Profit/Loss': [100, -200, 300, -400], 'Inventory Change': [-50, 60, -70, 80] } df_complex = pd.DataFrame(data) df_complex_abs = df_complex.abs() print(df_complex_abs)
Here, applying
abs()
is notably useful when analyzing the magnitude of changes and movements within financial or inventory data, irrespective of the direction (profit vs. loss or increase vs. decrease).
Combining with Other Functions for Enhanced Data Analysis
Synthesize
abs()
with other Pandas operations.Utilize conditional formatting for better visualization and interpretation.
pythondf_complex['Flag High Loss'] = (df_complex['Profit/Loss'] < -150).astype(int) df_analysis = df_complex.abs() print(df_analysis)
This example introduces a new column to flag significant losses. Usage of
abs()
helps to quickly convert values while additional conditions or computations can be appended to address specific analytical needs.
Practical Examples of abs() in Different Scenarios
Handling Inconsistencies in Data
Prepare a dataset with inconsistencies.
Use
abs()
to standardize the data representation.pythondf_inconsistent = pd.DataFrame({ 'Growth Rate': ['100%', '-200%', '300%', '-150%'] }) df_inconsistent['Growth Rate'] = df_inconsistent['Growth Rate'].str.replace('%', '').astype(int) df_inconsistent['Growth Rate'] = df_inconsistent['Growth Rate'].abs() print(df_inconsistent)
The conversion process involves removing percentage signs and converting string types to integers. Utilizing
abs()
then ensures all rates are expressed as positive values, facilitating more straightforward comparisons and computations.
Correlation Studies
Create a DataFrame with both positive and negative correlations.
Apply
abs()
to correlate data irrespective of the nature of the relationship.pythondata_analysis = { 'Variable1': [1, 2, 3, 4], 'Variable2': [-1, -2, -3, -4] } df_correlation = pd.DataFrame(data_analysis) correlation_matrix = df_correlation.corr().abs() print(correlation_matrix)
In correlation studies, the absolute values from
abs()
aid in understanding the strength of relationships without being biased by the direction of the correlation, making it helpful for preliminary data explorations.
Conclusion
The abs()
function in Pandas provides a straightforward method to convert all numerical values within a DataFrame to their absolute values. By utilizing this function in various data manipulation and analysis scenarios, standardize data formats, flag specific conditions, and simplify several analytical processes. These examples help demonstrate how the integration of abs()
into data workflows can enhance clarity and efficiency, ensuring that the magnitudes of changes and values are accessible and comparable across diverse datasets and analytical requirements.
No comments yet.