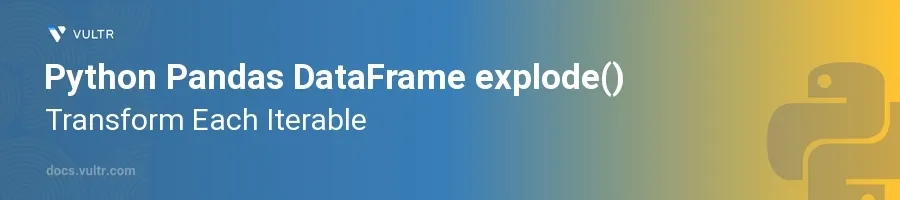
Introduction
The explode()
function in the Python Pandas library is a highly effective tool for transforming each iterable or list-like element in a DataFrame into separate rows. This function simplifies the process of dealing with nested data structures by flattening them out across multiple rows, thereby making data more accessible and easier to analyze. It's especially useful in scenarios where you have data that includes lists or arrays as column values.
In this article, you will learn how to leverage the explode()
function to enhance your data manipulation workflows. Understand how to apply this function to different types of data in your DataFrame to expand lists into new rows and explore practical examples that demonstrate the utility of explode()
in real-world data scenarios.
Exploding Lists in DataFrames
Basic Exploding of a Single Column
Create a DataFrame containing a column with list-like entries.
Use the
explode()
function on that specific column.pythonimport pandas as pd df = pd.DataFrame({ 'A': [[1, 2, 3], ['a', 'b'], [10]], 'B': [1, 2, 3] }) exploded_df = df.explode('A') print(exploded_df)
In this snippet, every list in column 'A' gets expanded into individual rows. The elements in each list become separate rows, with all other column values repeated for these new rows.
Exploding Multiple Columns Simultaneously
Start with a DataFrame where multiple columns contain iterables.
Apply
explode()
to each of these columns one by one.pythondf = pd.DataFrame({ 'A': [[1, 2], ['a', 'b']], 'B': [['x', 'y'], ['p', 'q']] }) exploded_df = df.apply(pd.Series.explode) print(exploded_df)
This approach applies the
explode()
function across all specified columns individually, transforming each iterable into multiple rows, aligning the exploded entries across columns.
Handling Nested and Composite Data
Exploding Nested Lists
Address nested lists within DataFrame columns that require multiple layers of exploding.
Execute a sequence of
explode()
calls.pythondf = pd.DataFrame({ 'Nested': [[[1, 2], [3, 4]], [[5, 6], [7, 8]]] }) first_explode = df['Nested'].explode() fully_exploded = first_explode.explode() print(fully_exploded.reset_index(drop=True))
This code takes a DataFrame with deeply nested lists and first explodes the outer list, then the inner lists, effectively flattening all levels of the nested structure into a single series.
Exploding and Filtering Data
Utilize
explode()
in conjunction with other Pandas operations like filtering to refine your results.Filter results based on conditions post-explode.
pythondf = pd.DataFrame({ 'Names': [['Alice', 'Bob', 'Charlie'], ['Dave', 'Eve']], 'Scores': [[85, 90, 75], [88, 92]] }) exploded_df = df.explode('Names') high_scores = exploded_df[exploded_df['Scores'] > 80] print(high_scores)
Here, after using
explode()
on the 'Names' column, a filtering condition is applied to select only the rows where 'Scores' exceed 80. This demonstrates howexplode()
can be integrated with other data transformation steps to target specific data insights.
Conclusion
The explode()
function in Pandas is instrumental in transforming columns containing lists or other iterable structures into individual rows, making complex data structures much more manageable and analysis-friendly. By mastering this function, you can handle various data manipulation tasks with ease, from expanding nested lists to integrating with sophisticated filtering and transformation operations. Employ the techniques and methods discussed here to streamline your data processing and enhance the clarity and accessibility of your datasets.
No comments yet.