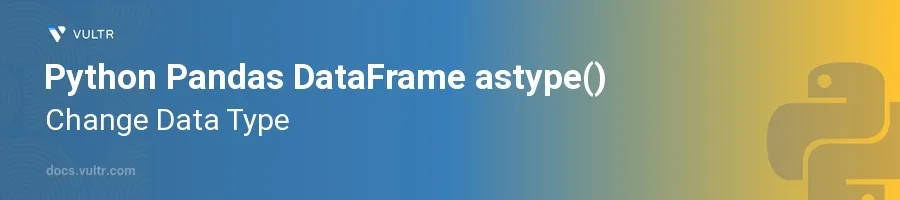
Introduction
Pandas is a powerful library in Python widely used for data manipulation and analysis, particularly with structured data like tables. One common need during data processing is converting the data types of DataFrame columns—this can be essential for ensuring correct data handling, especially when preparing data for machine learning models or data visualization tools. The astype()
method in Pandas is a versatile tool for such type conversions.
In this article, you will learn how to effectively utilize the astype()
method for changing data types in a Pandas DataFrame. Explore practical examples that demonstrate the method's application on various data types including integers, floats, and categorical data. These examples will help solidify your understanding of data type transformations in Pandas.
Understanding DataFrame's astype() Method
astype()
function is used to cast a pandas object to a specified dtype. It comes in handy when you need to make explicit data type conversions.
Basic Usage of astype()
Start with a simple DataFrame.
Convert the data type of one or more columns using
astype()
.pythonimport pandas as pd data = {'col1': [1, 2, 3], 'col2': ['4', '5', '6']} df = pd.DataFrame(data) df['col2'] = df['col2'].astype(int) print(df)
This code converts the
col2
from string type to integer type. The operation is performed in-place, directly modifying the data frame.
Converting Multiple Columns
Consider a DataFrame with several columns of different types.
Use
astype()
to alter multiple columns at once.pythondata = {'col1': [1, 2, 3], 'col2': ['4', '5', '6'], 'col3': [7.7, 8.8, 9.9]} df = pd.DataFrame(data) df = df.astype({'col1': 'float64', 'col2': 'int32'}) print(df)
In this example,
col1
is converted to float64 andcol2
to int32. The method allows for a dictionary to specify target data types for multiple columns, simplifying type conversion in complex DataFrames.
Practical Applications of astype()
Handling Missing Values
Consider null values in your DataFrame.
Convert data types while handling nulls appropriately.
pythonimport numpy as np data = {'col1': [1, 2, np.nan], 'col2': ['3', '4', '5']} df = pd.DataFrame(data) df['col1'] = df['col1'].astype('float64').fillna(0) df['col2'] = df['col2'].astype('int') print(df)
This code snippet ensures that the NaN values in
col1
are filled with 0 before conversion to prevent any type errors. Thecol2
is converted from string to integer directly.
Use of astype() for Categorical Data
Manage categorical data effectively.
Convert a column to categorical type to optimize memory usage.
pythondata = {'col1': ['apple', 'orange', 'banana', 'apple']} df = pd.DataFrame(data) df['col1'] = df['col1'].astype('category') print(df['col1'].cat.categories)
Converting a string column to a categorical type not only optimizes memory but also sets the groundwork for using pandas' powerful categorical methods, like accessing
.cat
attributes.
Conclusion
Mastering the astype()
method in Pandas significantly enhances your ability to manipulate data types within DataFrames. This tool is key for pre-processing steps in data analysis, ensuring that data types are correct for various computations, reducing memory usage, and potentially speeding up processing times. Apply these transformations for more efficient data manipulation and to leverage Pandas' full capability in handling diverse data types. Through the examples shared, acquire the confidence to apply .astype()
in various data scenarios, ensuring your datasets are optimally prepared for any analysis or processing workflow.
No comments yet.