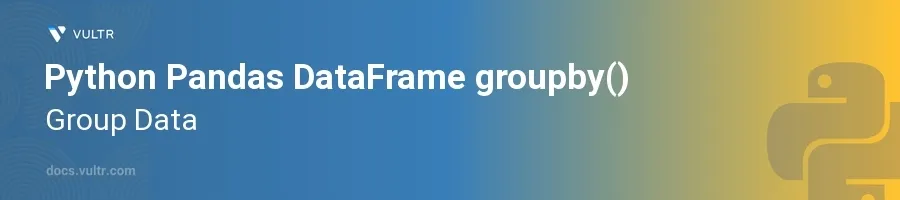
Introduction
The groupby()
function in Python pandas is an incredibly powerful tool for data aggregation, segmentation, and transformation. This function allows you to group large data sets by specific criteria, paving the way for more detailed and complex data analysis operations. Whether you are preparing data for analysis or aggregating results from multiple sources, groupby()
becomes indispensable in the toolkit of anyone working with data structured in DataFrame formats.
In this article, you will learn how to use the groupby()
function effectively to orchestrate and simplify data handling tasks. Explore practical examples to group data in different scenarios, understand how to apply aggregation functions, and learn techniques to transform grouped data for insightful analysis.
Basic Grouping in Pandas
Group by Single Column
Import the pandas library and create a DataFrame.
Use
groupby()
on a single column to see how data is split based on unique column values.pythonimport pandas as pd data = { 'Product': ['Apples', 'Oranges', 'Bananas', 'Apples', 'Oranges', 'Bananas'], 'Sales': [20, 35, 10, 15, 40, 25] } df = pd.DataFrame(data) grouped = df.groupby('Product')
After grouping,
grouped
is a DataFrameGroupBy object, not a regular DataFrame. It represents a group of DataFrame according to the unique values in 'Product'.
Observing Grouped Data
Use the
first()
,last()
, orget_group()
method to inspect elements of each group.pythonprint(grouped.first()) # shows the first entry of each group print(grouped.get_group('Apples')) # displays all entries under 'Apples'
These methods help to understand the organization of data in each group, showing how the
groupby()
function segregates the data based on the provided column.
Applying Aggregation
Summarize Data with Aggregations
Apply aggregation functions like
sum()
,mean()
, ormin()
to compute statistics for each group.pythontotals = grouped.sum() print(totals)
This code calculates the total sales for each product. Aggregation is one of the key aspects of grouping, allowing for a quick calculation of statistics across a dataset divided by specific categories.
Advanced Grouping Techniques
Multiple Columns Grouping
Group by more than one column to drill down into detailed data splits.
Use aggregation to explore combined statistics.
pythondata['Year'] = [2019, 2019, 2019, 2020, 2020, 2020] df = pd.DataFrame(data) grouped = df.groupby(['Year', 'Product']) summary = grouped.sum() print(summary)
Grouping by multiple columns gives a multi-index DataFrame, providing insights into hierarchical structures in the dataset, such as yearly and product-wise distribution of sales.
Custom Aggregation Functions
Define a custom aggregation function.
Apply it to the grouped data to cater to specific analytical needs.
pythondef range_func(group): return group.max() - group.min() range_sales = grouped['Sales'].agg(range_func) print(range_sales)
Custom functions can be used when built-in aggregations do not meet the requirements. It provides flexibility, allowing specific mathematical operations tailored to the analysis needs.
Transforming Groups
Apply Transformations to Groups
Use
transform()
to apply a function to each group while retaining the shape of the original DataFrame.pythonstandardized = grouped['Sales'].transform(lambda x: (x - x.mean()) / x.std()) print(standardized)
Transformation is useful when normalization or standardization of data within groups is required. It applies the function to each group separately but integrates the results back into the original DataFrame structure.
Conclusion
Harness the power of pandas' groupby()
function to simplify the management and analysis of grouped data. Whether for simple aggregations or complex grouped transformations, mastering this function elevates the data analysis capabilities to new heights. By implementing the techniques discussed, manage and analyze data more effectively, ensuring that insights derived from data are both meaningful and precise. Use this foundational knowledge to tackle more advanced data challenges, combining various pandas functions and methods to extract maximum value from your data.
No comments yet.