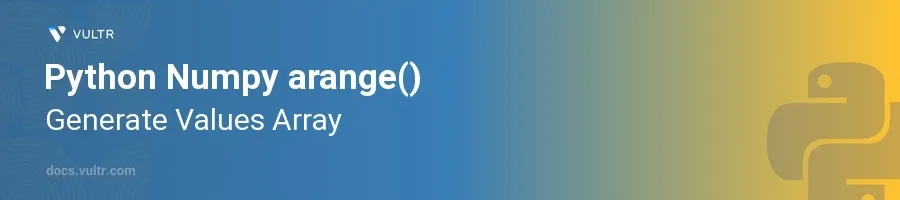
Introduction
The numpy.arange()
function in Python is a versatile tool for creating numerical sequences in arrays, essential in data manipulation and scientific computing. This function returns evenly spaced values within a given interval, similar to the range function but with enhancements that support floating point numbers and broader step increments.
In this article, you will learn how to skillfully utilize the numpy.arange()
function to generate arrays of values efficiently. Explore the different ways to fine-tune the output array using start, stop, and step parameters, and see how this function behaves with different data types.
Understanding arange() Function
Basic Usage of arange()
Import the Numpy library.
Use the
arange()
function to generate a simple array of integers.pythonimport numpy as np array_int = np.arange(10) print(array_int)
This code creates an array from 0 to 9. The function implicitly starts at 0 since no start value is specified and uses the default step of 1.
Specifying Start, Stop, and Step
Call
arange()
with start, stop, and step arguments to control the array values.Generate an array starting from 1, ending before 10, with a step of 2.
pythonarray_steps = np.arange(1, 10, 2) print(array_steps)
The output here considers a beginning at 1, stopping at 9 (since 10 is not inclusive), with increments of 2.
Handling Different DataTypes
Using Floats
Define
arange()
with floating point numbers to create non-integer sequences.Set up an array starting from 0, ending before 1, with a step of 0.1.
pythonarray_floats = np.arange(0, 1, 0.1) print(array_floats)
This demonstrates how
arange()
seamlessly handles floats, yielding a sequence from 0 to just below 1 with steps of 0.1.
Advanced Usage Scenarios
Generating a Date Range
Recognize that
arange()
can also handle dates using NumPy's datetime64 data type.Create an array of consecutive dates starting from a specific day.
pythondates_array = np.arange(np.datetime64('2023-01-01'), np.datetime64('2023-01-10')) print(dates_array)
This setup creates an array of dates from January 1, 2023, to January 9, 2023.
Conclusion
The numpy.arange()
function provides a simple yet powerful way to generate arrays of numbers in Python. It's beneficial for creating sequences with specific intervals, handling different data types like integers, floats, and dates with ease. By mastering the arange()
function, ensure efficient manipulation in numerical computing and array generation to streamline your data processing tasks.
No comments yet.