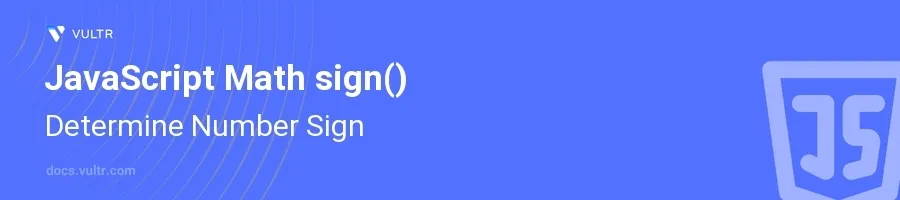
Introduction
The Math.sign()
function in JavaScript is an invaluable tool for determining the sign of a number, returning 1, -1, 0, -0, or NaN depending on the sign of the given value. This utility function facilitates operations such as data validation, conditional rendering, and numerical computations where the numerical sign affects the logic or outcome.
In this article, you will learn how to effectively use the Math.sign()
function in various programming scenarios. Explore how this function aids in handling different numerical inputs and the potential use cases in JavaScript applications.
Understanding Math.sign()
Basic Usage of Math.sign()
Pass a positive number to receive a positive sign.
javascriptconsole.log(Math.sign(3)); // Output: 1
This code returns
1
indicating the number is positive.Pass a negative number to see a negative outcome.
javascriptconsole.log(Math.sign(-3)); // Output: -1
This result of
-1
denotes a negative number.
Handling Zero and Negative Zero
Understand how
Math.sign()
handles zero.Pass zero to
Math.sign()
to see the behavior.javascriptconsole.log(Math.sign(0)); // Output: 0 console.log(Math.sign(-0)); // Output: -0
The function differentiates between
0
and-0
. This distinction is crucial for specific computational scenarios.
Evaluating Non-Number Types
Pass a non-numeric value to see how
Math.sign()
handles it.Use a string representation of a number to test conversion capabilities.
javascriptconsole.log(Math.sign('10')); // Output: 1 console.log(Math.sign('')); // Output: 0 console.log(Math.sign('abc')); // Output: NaN
Math.sign()
attempts to convert strings to numbers. It returns1
for '10' as it's a positive number,0
for an empty string, andNaN
for non-numeric strings.
Practical Applications of Math.sign()
Conditional Logic Enhancement
Integrate
Math.sign()
in if-else structures to simplify conditions.javascriptlet score = -5; let label = Math.sign(score) > 0 ? "Positive" : "Negative"; console.log(label); // Output: Negative
This approach minimizes the complexity of conditional checks by relying on the sign of the number.
Array Sorting Based on Sign
Use
Math.sign()
for custom sorting in arrays.javascriptlet numbers = [3, -2, 0, -1, 4]; numbers.sort((a, b) => Math.sign(b) - Math.sign(a)); console.log(numbers); // Output: [3, 4, 0, -2, -1]
This snippet sorts numbers by their sign, positioning positive numbers first, followed by zeros, then negatives.
Conclusion
The Math.sign()
function in JavaScript is an effective utility for determining the sign of numerical values. Its application extends beyond simple sign determination; it aids in handling validations, sorting algorithms, and implementing conditional logic based on numerical values. Harness the simplicity and power of Math.sign()
to make your JavaScript code cleaner and more efficient, while managing numerical data with ease.
No comments yet.