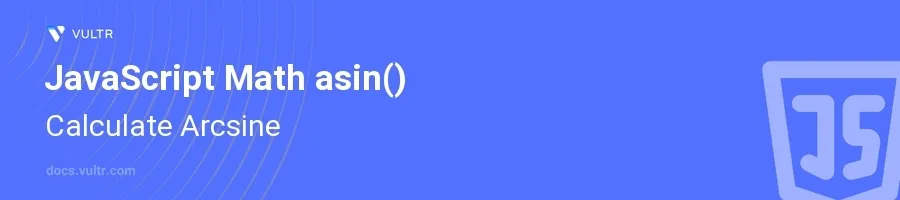
Introduction
The Math.asin()
function in JavaScript is used to calculate the arcsine (inverse sine) of a number. This mathematical function is essential when dealing with trigonometric calculations, especially when converting from a sine value back to an angle. It can be used in various applications such as physics simulations, graphical computations, and any scenario where angle determination is essential.
In this article, you will learn how to use the Math.asin()
function in JavaScript for different types of inputs. You will explore examples that demonstrate how to compute the arcsine of both valid and invalid inputs, and how to handle these cases appropriately in your code.
Calculating Arcsine with Valid Inputs
Compute Arcsine of Zero
Use
Math.asin()
with an argument of zero to find the arcsine.Display the result using
console.log
.javascriptconst result = Math.asin(0); console.log(result); // Output: 0
The arcsine of zero yields an angle of zero radians.
Calculate Arcsine for Typical Sine Values
Declare a variable with a sine value for which you know the corresponding angle.
Apply the
Math.asin()
method and print the results.javascriptlet value = 0.5; const angle = Math.asin(value); console.log(angle); // Output: 0.5235987755982989
Here,
0.5
is the sine of 30 degrees or approximatelyπ/6
radians. This example confirms the angle is calculated correctly.
Handling Edge Cases
Using Out-of-Range Values
Test
Math.asin()
with a value that exceeds the valid input range (-1 to 1
).Observe and display the result.
javascriptconst outOfRange = Math.asin(1.5); console.log(outOfRange); // Output: NaN
This code highlights how
Math.asin()
handles values outside the-1
to1
range, returningNaN
for such cases.
Application with Negative Values
Understand that the arcsine function can also process negative values within the acceptable range.
Call
Math.asin()
with a negative input and print the output.javascriptconst negativeResult = Math.asin(-0.5); console.log(negativeResult); // Output: -0.5235987755982989
The function returns
-π/6
radians for-0.5
, which is the negative equivalent of the 30 degrees calculated earlier.
Conclusion
The Math.asin()
function in JavaScript is invaluable for computing the arcsine of a given sine value, which is essential in many fields involving trigonometric calculations. It provides a straightforward method to derive angles from sine values and efficiently handles cases through its defined input range of -1
to 1
. By following the practices and examples presented, handling mathematical calculations in trigonometric contexts within JavaScript applications becomes more accessible and accurate. Ensure to always check input values to maintain the accuracy and reliability of your computations.
No comments yet.