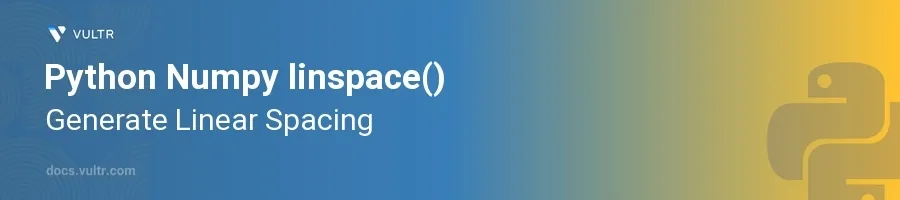
Introduction
The linspace()
function from the NumPy library in Python provides an efficient and easy way to generate linearly spaced values within a specified range. This function is crucial for creating datasets for simulations, graphs, and mathematical computations where specific intervals between numbers are necessary.
In this article, you will learn how to utilize the linspace()
function in various scenarios. Discover how this function simplifies the process of generating evenly spaced values, and explore practical examples to fully grasp its application in data analysis and mathematical modeling.
Generating Evenly Spaced Numbers
Basic Usage of linspace()
Specify the start and end values of the range.
Determine the number of evenly spaced points needed between the start and end.
Call the
linspace()
function with these parameters.pythonimport numpy as np linear_space = np.linspace(1, 10, 10) print(linear_space)
This code creates an array of 10 linearly spaced points between 1 and 10, inclusive.
Customizing Endpoint Inclusion
Understand that
linspace()
includes the endpoint by default, but it can be excluded.Utilize the
endpoint
parameter to control this behavior.Generate an array excluding the endpoint.
pythonlinear_space_no_endpoint = np.linspace(0, 1, 5, endpoint=False) print(linear_space_no_endpoint)
Here,
linspace()
generates 5 evenly spaced points between 0 and 1, not including 1.
Non-integer Spacing
Realize that
linspace()
supports generating points with non-integer steps.Generate an array where non-integer spacing is desired.
pythonnon_integer_space = np.linspace(0, 1, 6) print(non_integer_space)
The example demonstrates generating values between 0 and 1 with non-integer spacing.
Advanced Features of linspace()
Controlling Output Data Type
Set the
dtype
parameter to specify the data type of the output array.Create an array of specific type, such as integers or floats.
pythoninteger_space = np.linspace(0, 10, 5, dtype=int) print(integer_space)
By specifying
dtype=int
,linspace()
creates an array of integers instead of the default floats.
Using linspace() for Complex Numbers
Utilize
linspace()
to generate linear spaces of complex numbers.Define start and end points as complex numbers to do this effectively.
pythoncomplex_space = np.linspace(1+1j, 10+10j, 10) print(complex_space)
This snippet generates 10 linear steps from
1+1j
to10+10j
, showinglinspace()
's versatility with complex numbers.
Conclusion
NumPy's linspace()
function is a valuable tool for generating evenly spaced sequences of numbers. Its ability to customize the inclusion of the endpoint, specify the data type, and work with complex numbers allows for flexibility across various mathematical and simulation tasks. Applying the linspace()
function as demonstrated helps streamline data generation processes, enabling more efficient and precise computations and visualizations. By mastering this function, improve the clarity and performance of numerical simulations and data analysis endeavors.
No comments yet.