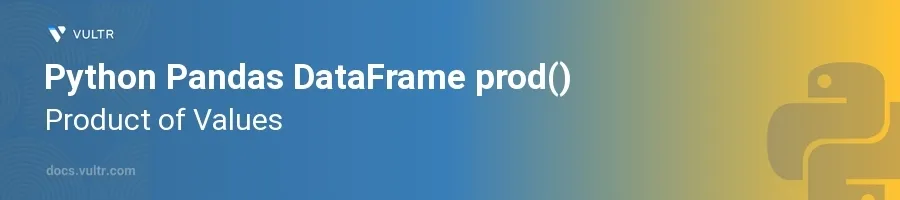
Introduction
The prod()
method in Pandas is a powerful tool for calculating the product of numerical data across a DataFrame or a Series. This function is central in statistics and data analysis, especially when determining the cumulative product of values in datasets related to finance, physics, or any domain where multiplication aggregations are meaningful. Efficient and versatile, prod()
simplifies multiplicative operations over arrays of data, facilitating more complex mathematical computations.
In this article, you will learn how to effectively employ the prod()
method on Pandas DataFrames. Discover how to compute the product of entire datasets or selective portions, handle missing values, and manipulate the axis parameter to tailor results specific to your data analysis needs.
Applying prod() on Entire DataFrame
Calculate the Product of All Values
Initialize a DataFrame with numeric values.
Apply the
prod()
method to compute the product of all the values.pythonimport pandas as pd data = {'A': [2, 3, 4], 'B': [5, 6, 7]} df = pd.DataFrame(data) total_product = df.prod().prod() print(total_product)
This script creates a DataFrame
df
from a dictionary of lists and calculates the product of all values across the DataFrame. The firstprod()
method computes the product in each column, and the secondprod()
computes the product of these results.
Understanding the Output
- The product for column 'A' is 24 (2 * 3 * 4).
- The product for column 'B' is 210 (5 * 6 * 7).
- The final product across the entire DataFrame is 5040 (24 * 210).
Computing Column-specific Products
Calculate Product along an Axis
Organize your data such that each column is a variable of interest.
Utilize the
prod()
method setting theaxis
parameter to 0 to compute the product along the columns.pythoncolumn_product = df.prod(axis=0) print(column_product)
This function calculates the product of the values for each column separately, treating each column as an independent array of numbers.
Axis Details
- Setting
axis=0
computes the product down each column. - For operations across rows, set
axis=1
.
Handling Missing Data with prod()
Working with NaN Values
Add missing values to your DataFrame.
Apply
prod()
and observe its handling ofNaN
.pythondf.loc[2, 'B'] = None # Introduce a NaN value nan_product = df.prod(axis=0, min_count=1) print(nan_product)
By setting
min_count=1
, the product calculation will proceed even if there's only one non-NaN value. This is useful for ensuring that the presence of missing data doesn't entirely impede your product calculations.
Explanation of NaN Handling
- Pandas typically treats
NaN
as 'no value', thus a product operation involvingNaN
would normally result inNaN
. - The
min_count
parameter defines the minimum number of valid values required. If the data available reaches this threshold, the calculation considers those values.
Manipulating Product Calculations with Skipna
Excluding NaNs from Calculations
Ensure your DataFrame contains some missing values.
Use the
skipna
option in theprod()
method to control whether to include or excludeNaN
.pythonskipna_product = df.prod(axis=0, skipna=True) print(skipna_product)
By setting
skipna=True
, all NaN values are excluded, allowing the product calculation only over available, valid numbers, which helps in reports or statistical analysis where NaN signifies lack of data rather than zero.
Conclusion
The prod()
method in Pandas is invaluable for comprehensive multiplicative aggregation of dataset values. Whether you're looking to compute the product of entire dataframes, specific columns, or even manage datasets with missing values, the prod()
method provides robust options to handle various data complexities. Employ this method to streamline data transformations and extend numerical analyses in your projects, ensuring you deliver precise and meaningful statistical interpretations.
No comments yet.