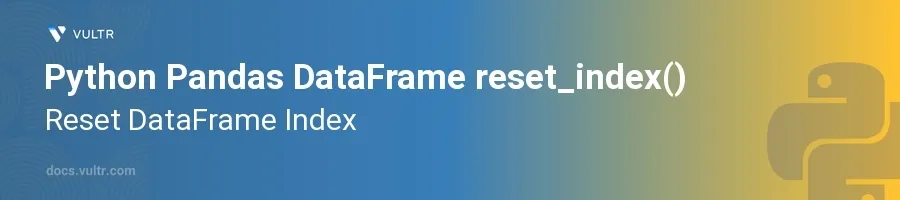
Introduction
Handling data effectively in Python often involves the use of Pandas DataFrames, a powerful tool for data manipulation and analysis. One common task you might face while working with DataFrames is resetting their index. The reset_index()
method is crucial when you need to transform the index of your DataFrame into a more suitable format after operations like sorting, filtering, or subsetting have altered the structure of the DataFrame.
In this article, you will learn how to utilize the reset_index()
method in various contexts. Explore how to reset the index to the default integer index, work with the drop
and inplace
parameters to modify the DataFrame directly, and understand how to incorporate the previous index as a column in the DataFrame.
Understanding the Basics of reset_index()
Resetting the DataFrame Index
Start with a simple DataFrame.
Use
reset_index()
to reset the index.pythonimport pandas as pd df = pd.DataFrame({ "A": [1, 2, 3], "B": [4, 5, 6] }, index=['x', 'y', 'z']) reset_df = df.reset_index() print(reset_df)
This example starts with a DataFrame
df
that has a custom index ('x', 'y', 'z'). Thereset_index()
method resets the DataFrame's index to the default integer index, and the original index becomes a new column in the DataFrame.
Using the drop
Parameter
Understand the purpose of the
drop
parameter, which removes the existing index and does not add it as a column in the DataFrame.Implement
reset_index()
withdrop=True
.pythondropped_index_df = df.reset_index(drop=True) print(dropped_index_df)
In this case,
drop=True
tells Pandas not to retain the old index. After usingreset_index()
, the original index is discarded, and the DataFrame retains only its data columns.
Specifying the inplace
Parameter
Learn about
inplace
, which allows the modifications to affect the original DataFrame without creating a new object.Apply
reset_index()
withinplace=True
.pythondf.reset_index(drop=True, inplace=True) print(df)
Applying
inplace=True
in combination withdrop=True
modifiesdf
directly, resetting its index in place and discarding the old index, sodf
itself is updated without needing to reassign it to a new variable.
reset_index() in Multilevel Index DataFrames
Multi-Index DataFrame Example
Recognize the use of
reset_index()
in DataFrames with multiple levels of indexing.Demonstrate resetting the level and how to handle multiple indices.
pythonarrays = [['bar', 'bar', 'baz', 'baz'], ['one', 'two', 'one', 'two']] index = pd.MultiIndex.from_arrays(arrays, names=['first', 'second']) df_multi = pd.DataFrame({ "A": [1, 2, 3, 4], "B": [5, 6, 7, 8] }, index=index) multi_reset = df_multi.reset_index() print(multi_reset)
In multi-index scenarios, the
reset_index()
method converts each level of the index into separate columns. The resulting DataFrame has a default numerical index and includes the former index levels as columns.
Resetting Specific Levels
Understand how to specify levels to reset in multi-index.
Reset only certain levels using the
level
parameter.pythonpartial_reset = df_multi.reset_index(level='second') print(partial_reset)
This code snippet resets only the 'second' level of the index. The 'first' level remains intact as part of the DataFrame's index. This flexibility allows precise control over which parts of the index are turned into columns.
Conclusion
The reset_index()
function in Python's Pandas library is a versatile tool for managing DataFrame indices. Whether handling simple, single-level indexes, or navigating the complexities of multi-level indexing, this method provides a straightforward approach for reformatting and manipulating DataFrame indices. Use reset_index()
to make your DataFrames easier to work with, especially after operations that modify the DataFrame structure.
No comments yet.