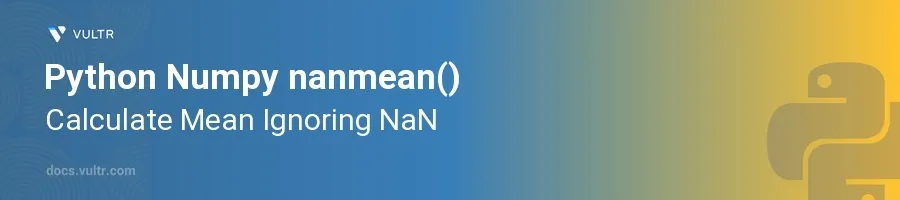
Introduction
The numpy.nanmean()
function in Python is a specific tool used to calculate the arithmetic mean of an array, ignoring all NaN
(Not a Number) values. This function is crucial when dealing with datasets that contain missing or undefined values, allowing for more accurate statistical analyses without the need to manually clean the data first.
In this article, you will learn how to adeptly utilize the numpy.nanmean()
function to compute means in various scenarios. Explore its application in single-dimensional and multi-dimensional arrays, and see how it behaves with different data types.
Calculating Mean in Single-Dimensional Arrays
Calculate Mean Excluding NaNs
Import the Numpy library.
Create a one-dimensional numpy array containing some
NaN
values.Use the
numpy.nanmean()
function to compute the mean while skippingNaN
values.pythonimport numpy as np data = np.array([1, 2, np.nan, 4, 5]) mean_value = np.nanmean(data) print(mean_value)
This script calculates the mean of the array while ignoring the
NaN
value. The output is the mean of the available (non-NaN
) numbers.
Handling Arrays with All NaNs
Understand that a completely
NaN
dataset can lead to undefined results.Prepare such an array and apply
nanmean()
.pythonall_nan_data = np.array([np.nan, np.nan, np.nan]) mean_all_nan = np.nanmean(all_nan_data) print(mean_all_nan)
Here, because all elements are
NaN
,numpy.nanmean()
returnsnan
as the result, symbolizing an undefined mean.
Working with Multi-Dimensional Arrays
Compute Mean Across Different Axes
Recognize that
numpy.nanmean()
can operate across different axes of a multi-dimensional array.Construct a two-dimensional array with
NaN
values.Apply
numpy.nanmean()
specifying the axis.pythonmulti_data = np.array([[7, np.nan, 3], [np.nan, 5, 1]]) mean_axis0 = np.nanmean(multi_data, axis=0) mean_axis1 = np.nanmean(multi_data, axis=1) print("Mean across axis 0 (columns):", mean_axis0) print("Mean across axis 1 (rows):", mean_axis1)
This code computes the mean across columns (
axis=0
) and rows (axis=1
), respectively, effectively handlingNaN
values.
Conclusion
The numpy.nanmean()
function is essential for statistical computing, especially in datasets plagued with NaN
entries. Its ability to gracefully omit NaN
values from calculations prevents the distortion of statistical results, rendering it invaluable for robust data analysis. Leveraging numpy.nanmean()
in single-dimensional and multi-dimensional contexts ensures that analyses remain accurate and meaningful by adequately dealing with incomplete data. Advanced handling of entire NaN
arrays further empowers users to maintain well-defined analytical procedures across diverse datasets.
No comments yet.