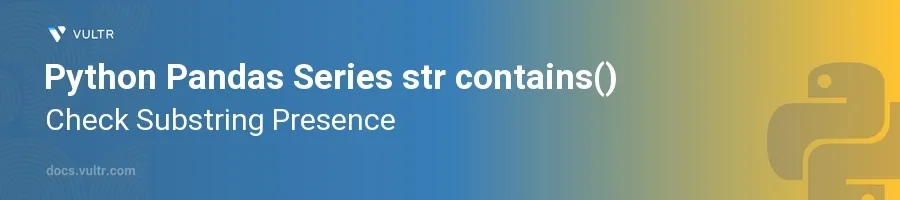
Introduction
The str.contains()
method in Pandas is an essential tool for checking the presence of a substring within each string element of a Series. This approach is particularly useful in data analysis and processing where conditions based on string patterns need to be evaluated. For instance, filtering data rows based on textual content, checking for compliance in datasets, or simply categorizing strings can all leverage this functionality.
In this article, you will learn how to efficiently use the str.contains()
method in various data manipulation scenarios. Understand how this method facilitates string pattern matching in a Pandas Series and discover practical examples that demonstrate its application in real world data tasks.
Utilizing str.contains() in Data Filtering
Basic Usage of str.contains()
Import the necessary libraries and create a Pandas Series.
Apply the
str.contains()
method to find substrings in the series.pythonimport pandas as pd data = pd.Series(['apple', 'banana', 'cherry', 'date']) mask = data.str.contains('a') print(mask)
The code above creates a series of fruit names and then uses
str.contains()
to find all entries containing the letter 'a'. The result is a boolean series indicating which elements match the condition.
Case Sensitivity Management
Handle case sensitivity by adjusting the
case
parameter.Demonstrate how toggling this parameter affects string matching.
pythonmask = data.str.contains('A', case=False) print(mask)
This example considers both uppercase and lowercase letters by setting
case
toFalse
. It outputs a boolean series where every instance of 'a' or 'A' leads to aTrue
value.
Complex Patterns with Regular Expressions
Use regular expressions for more complex substring matching.
Build a pattern that matches more than one condition.
pythonmask = data.str.contains('a|e', regex=True) print(mask)
Here,
str.contains()
analyzes the series to find any occurrence of 'a' or 'e'. The|
character in the regular expression denotes a logical OR, so any string containing either character is flagged asTrue
.
Filtering Data Frames
Create a DataFrame with textual data.
Employ
str.contains()
to filter the DataFrame based on string patterns.pythondf = pd.DataFrame({ 'Fruit': ['apple', 'banana', 'cherry', 'date'], 'Color': ['red', 'yellow', 'red', 'brown'] }) filtered_df = df[df['Fruit'].str.contains('a')] print(filtered_df)
In this scenario, a DataFrame is filtered by the
Fruit
column where the presence of 'a' determines which rows are included in the result.
Advanced Usage Scenarios
Handling Missing Values
Recognize how
str.contains()
behaves when encountering NaN values.Utilize the
na
parameter to handle missing data gracefully.pythondata_with_na = pd.Series(['apple', 'banana', None, 'date']) mask = data_with_na.str.contains('a', na=False) print(mask)
With missing data points (NaN values), setting
na=False
ensures that these are treated asFalse
in the resulting Boolean mask, avoiding errors during filtering.
Working with Flags in Regular Expressions
Incorporate flags to extend the functionality of regex patterns.
Use a flag to handle case-insensitive matching in regex.
pythonmask = data.str.contains('A|B', regex=True, flags=re.IGNORECASE) print(mask)
By introducing
flags=re.IGNORECASE
from there
library, you enhance the regular expression to ignore case sensitivity.
Conclusion
The str.contains()
function in Python Panda Series offers a powerful yet straightforward method to check for the presence of substrings, allowing you to filter and manage data effectively. Whether dealing with simple substring checks or complex pattern recognitions, this function simplifies the approach to string matching in large datasets, ensuring that data analytics tasks are not only feasible but efficient. By implementing the techniques discussed, maximize the insights derived from your textual data while maintaining a clean and efficient codebase.
No comments yet.