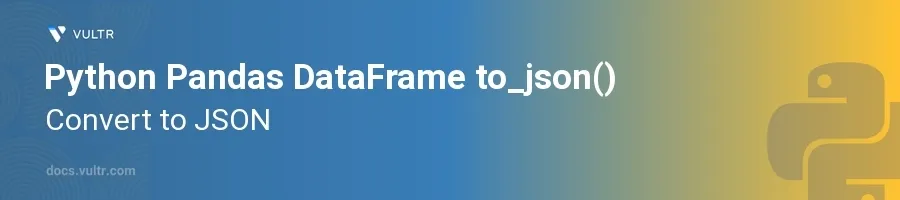
Introduction
The to_json()
method in Python's Pandas library is an efficient tool for converting DataFrame structures into JSON format. This capability is particularly valuable in data interchange scenarios where JSON is the preferred format for data transmission over web APIs, storage in NoSQL databases, or simply for human-readable files. The method offers a variety of customization options to tailor the output to specific needs.
In this article, you will learn how to effectively convert a DataFrame to JSON using the to_json()
method. Discover the various parameters that control the serialization process and how you can utilize them to format the JSON output according to your requirements. Mastering this function will enhance your ability to manage data exports and integration tasks seamlessly.
Understanding DataFrame to JSON Conversion
Converting a DataFrame to JSON involves calling the to_json()
method, which serializes the DataFrame object to a JSON string. This method supports different orientations which dictate how data is represented in JSON.
Basic Conversion
Prepare a DataFrame with sample data.
Convert the DataFrame to a JSON string using the default parameters.
pythonimport pandas as pd # Sample DataFrame data = {'Name': ['John', 'Anna'], 'Age': [28, 22]} df = pd.DataFrame(data) # Convert DataFrame to JSON json_data = df.to_json() print(json_data)
In this example, the DataFrame
df
is converted to a JSON string. By default, theto_json()
method uses the 'columns' orientation, where each column becomes a key, and their values are listed in an array.
Customizing the Output Format
Experiment with different orientations like
split
,records
,index
, andvalues
.Convert the DataFrame to JSON using these orientations and observe the outputs.
python# Using different orientations json_split = df.to_json(orient='split') print("JSON split format:", json_split) json_records = df.to_json(orient='records') print("JSON records format:", json_records) json_index = df.to_json(orient='index') print("JSON index format:", json_index) json_values = df.to_json(orient='values') print("JSON values format:", json_values)
Each orientation offers a unique structure:
split
: Serialized data into separate lists for indexes, columns, and data.records
: List of records, where each record is a JSON object.index
: JSON objects where each object key is its index in the DataFrame.values
: Only the values are serialized into arrays.
Handling Complex Data Types
DataFrame columns can contain complex data types, such as nested lists, dictionaries, or even other DataFrames. Handling these types requires attention to ensure they are appropriately serialized.
Serialize Nested Structures
Create a DataFrame containing complex data structures.
Convert to JSON and specify how to handle non-scalar types.
pythondf_complex = pd.DataFrame({ 'Name': ['John', 'Anna'], 'Details': [{'gender': 'male', 'age': 28}, {'gender': 'female', 'age': 22}] }) json_complex = df_complex.to_json() print(json_complex)
Here, non-scalar values in the
Details
column are converted into nested JSON objects, preserving the hierarchical structure.
Serialization Parameters
Pandas' to_json()
allows further customization through additional parameters that control date formatting, default handlers for unsupported data types, and more.
Adjust Parameters for Enhanced Control
Explore parameters like
date_format
,double_precision
, anddefault_handler
.Apply these parameters to fine-tune the JSON output.
pythondf_dates = pd.DataFrame({ 'Name': ['John', 'Anna'], 'Birthday': [pd.Timestamp('1988-05-15'), pd.Timestamp('1990-08-08')] }) json_dates = df_dates.to_json(date_format='iso') print("Date in ISO format:", json_dates)
The
date_format
parameter allows date and time to be formatted in a specific way, in this case, using the ISO 8601 format.
Conclusion
Using Pandas DataFrame's to_json()
function grants powerful capabilities for converting data into JSON, a format ubiquitous in data interchange contexts. By mastering different orientations and parameters, you ensure that the data export process is precise, suitable for various downstream applications, from API interactions to file storage. Implement these techniques to make data handling more efficient and integrated within your workflows, keeping your data pipelines robust and adaptable.
No comments yet.