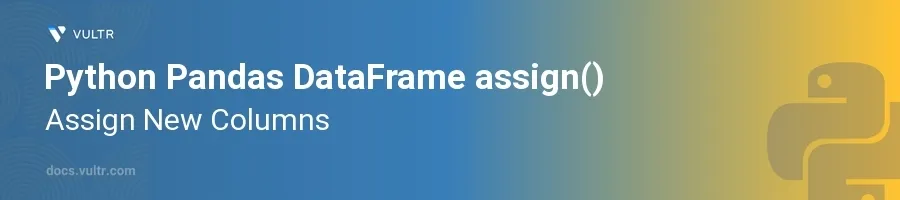
Introduction
The assign()
method in Pandas is a versatile tool for adding new columns to a DataFrame in a way that promotes readability and ease of use. This method is particularly useful in data transformation tasks where new derived columns are created from existing data or through external computations. The method returns a new DataFrame, leaving the original DataFrame untouched, which aligns with functional programming principles.
In this article, you will learn how to proficiently utilize the assign()
method to add new columns to a DataFrame. Explore various examples that demonstrate how this function seamlessly integrates with lambda functions and facilitates more complex data manipulations.
Understanding the assign() Method
Basic Usage of assign()
Start by importing Pandas and creating a simple DataFrame.
pythonimport pandas as pd df = pd.DataFrame({ 'A': range(1, 5), 'B': range(10, 50, 10) })
Use the
assign()
method to add a new column.pythondf_assigned = df.assign(C=lambda x: x['A'] + x['B']) print(df_assigned)
This code adds a new column
C
that is the sum of columnsA
andB
in the DataFrame. The lambda functionlambda x: x['A'] + x['B']
is applied row-wise.
Using Multiple Assignments
To add multiple columns, chain assignments within the same
assign()
call.pythondf_assigned = df.assign( C=lambda x: x['A'] + x['B'], D=lambda x: x['A'] * x['B'] ) print(df_assigned)
The
assign()
method can accept multiple lambdas which allows for the creation of multiple new columns in one streamlined operation.
Integrating Conditional Logic
Introduce conditions to dynamically assign values based on other column data.
pythondf_assigned = df.assign( Category=lambda x: ['High' if a > 2 else 'Low' for a in x['A']] ) print(df_assigned)
The
Category
column is calculated based on whether values in columnA
are greater than 2, demonstrating how to incorporate conditional logic into column assignments.
Advanced Data Manipulations with assign()
Handling Missing Data
Use the
assign()
method to replace missing data in a new column creation.pythondf_with_na = pd.DataFrame({ 'A': [1, 2, None, 4], 'B': [10, None, 30, 40] }) df_filled = df_with_na.assign( A_filled=lambda x: x['A'].fillna(0), B_filled=lambda x: x['B'].fillna(x['B'].mean()) ) print(df_filled)
This example deals with missing data by filling it with default values or mean of the existing values, showcasing another practical application of
assign()
in data preprocessing.
Using assign() with External Functions
Integrate external functions for more complex transformations.
pythondef calculate_complex_value(row): return row['A'] * 2 + row['B'] ** 2 df_assigned = df.assign( ComplexValue=calculate_complex_value ) print(df_assigned)
Here,
assign()
calls an external function,calculate_complex_value
, which performs a calculation using multiple columns and adds the results as a new column.
Conclusion
The assign()
method in Pandas greatly enhances data manipulation capabilities, providing an intuitive and powerful way to add new columns to DataFrames. It supports the use of lambda functions and external function integration, allowing for efficient transformations and complex calculations. By mastering assign()
, streamline your data processing workflows, ensuring that they are efficient, readable, and maintain functional programming principles. Adapt the examples given to match your specific data analysis needs and see how assign()
can simplify adding new columns and managing data transformations.
No comments yet.