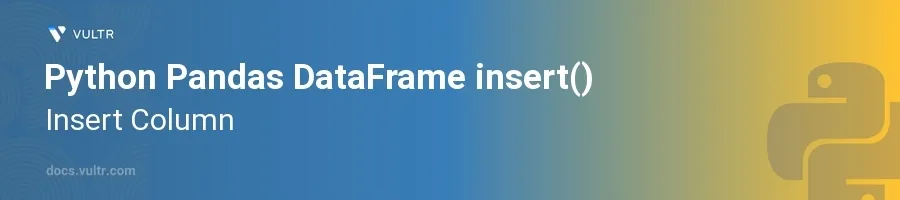
Introduction
The insert()
method in Pandas is a dynamic way to add a column at a specific location within a DataFrame. Whether you're rearranging data for a presentation, preparing data for analysis, or just manipulating data for better insight, adding columns precisely where you need them is crucial.
In this article, you will learn how to efficiently use the insert()
method. Explore various use cases such as inserting single-value columns, calculated columns based on existing data, and inserting columns with non-standard data types. By the end, you'll be able to enhance your DataFrames on-the-fly with this powerful feature.
Understanding the insert() Method
Basic Usage of insert()
Familiarize yourself with the function signature:
pythonDataFrame.insert(loc, column, value, allow_duplicates=False)
The parameters are:
- loc: The integer index indicating the position in the DataFrame to insert the new column.
- column: The string that will be used as the column name.
- value: The data to insert, which can be a scalar, a series, or an array.
- allow_duplicates: A boolean that allows duplicated column titles if set to True.
Example: Inserting a Simple Column
Create a sample DataFrame to work with.
Choose the insertion point and the data for the new column.
Use the
insert()
method to add the column.pythonimport pandas as pd # Sample DataFrame df = pd.DataFrame({ 'A': range(1, 6), 'B': range(10, 15) }) # Inserting a new column df.insert(1, 'NewColumn', range(100, 105)) print(df)
This block creates a DataFrame with columns 'A' and 'B'. The
insert()
function then adds 'NewColumn' between them with the given range.
Advanced Usage of insert()
Adding a Calculated Column
Define a new column that is a function of existing data.
Insert the new computed column at the desired position.
python# Calculation based on existing columns new_values = df['A'] * 2 + df['B'] df.insert(2, 'CalculatedColumn', new_values) print(df)
In this example, the new column 'CalculatedColumn' is calculated using the values from columns 'A' and 'B', and then it is inserted at position 2 in the DataFrame.
Conditional Insert
Suppose you want to insert a column based on a condition.
Use a conditional expression to generate the data and then insert.
python# Conditional Column Insert condition = df['A'] > 3 df.insert(3, 'Is_A_Greater_3', condition) print(df)
This will insert a new boolean column that tells whether each value in column 'A' is greater than 3.
Inserting Non-Standard Data Types
Inserting DateTime and Categorical Data
Generate data of non-standard types such as datetime or categorical.
Insert this data into the DataFrame.
pythonimport pandas as pd # Creating a sample DataFrame df = pd.DataFrame({ 'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve'] }) # Inserting datetime data date_series = pd.date_range('20230101', periods=5) df.insert(1, 'Date', date_series) # Inserting categorical data category_series = pd.Series(["Group A", "Group B", "Group A", "Group B", "Group A"], dtype="category") df.insert(2, 'Category', category_series) print(df)
This snippet adds both datetime and categorical columns to a DataFrame consisting initially of a single text column.
Conclusion
Mastering the insert()
method in Pandas enhances your ability to manipulate DataFrames effectively, making data re-organization, preparation, and analysis more intuitive and efficient. Whether it's adding simple static data, calculated values, or handling complex data types, the insert()
functionality accommodates various data manipulation needs. Apply these techniques to insert columns strategically within your DataFrames and optimize your data handling tasks in any Python data analysis project.
No comments yet.