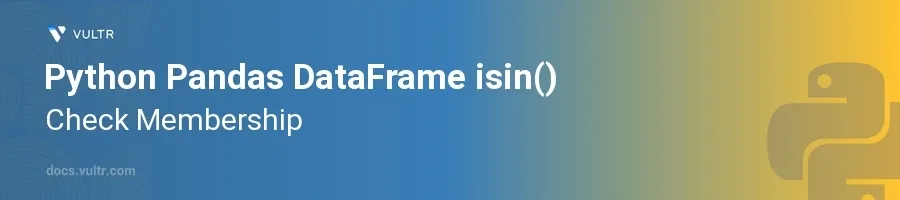
Introduction
The isin()
method in Python's Pandas library is a versatile tool for querying DataFrame objects to check for membership in a list, DataFrame, or Series. This utility proves essential in data analysis tasks, allowing for the filtering of data based on criteria, which can optimize your workflows and data interrogation processes significantly.
In this article, you will learn how to effectively use the isin()
method across different datasets. Explore practical strategies to ensure that your data wrangling becomes more efficient and straightforward, fully leveraging the capabilities of Pandas in Python.
Understanding the isin() Method
Basic Usage with a List
Start by importing Pandas and creating a DataFrame.
Define a list against which to check membership.
Use the
isin()
method on one or more DataFrame columns.pythonimport pandas as pd df = pd.DataFrame({ 'A': [1, 2, 3], 'B': ['a', 'b', 'c'] }) check_list = [1, 'a'] result = df.isin(check_list) print(result)
This code snippet creates a DataFrame with numbers and characters and checks each element's membership against
check_list
. Each DataFrame cell is evaluated independently, with a boolean value indicating membership.
Use in Filtering DataFrames
Create or import a DataFrame that contains real-world data.
Specify a list of values for which to check membership.
Apply the
isin()
method to filter the DataFrame based on the specified values.pythondata = { 'Product': ['Apple', 'Banana', 'Cherry'], 'Price': [80, 30, 90] } df = pd.DataFrame(data) prices_to_check = [80, 90] filtered_df = df[df['Price'].isin(prices_to_check)] print(filtered_df)
Here,
filtered_df
contains only the rows where the 'Price' column's values are either 80 or 90. This targeted data filtering is crucial for tasks like sales analysis or stock management.
Advanced Applications of isin()
Checking Against Another DataFrame or Series
Consider multiple DataFrames or Series that you wish to compare.
Utilize the
isin()
method to determine if values in one DataFrame exist in another, using a Series or DataFrame as the argument.pythondf1 = pd.DataFrame({ 'Key': ['A', 'B', 'C', 'D'] }) df2 = pd.DataFrame({ 'Ref': ['A', 'E', 'I', 'O', 'U'] }) df1['Exists_in_DF2'] = df1['Key'].isin(df2['Ref']) print(df1)
The resulting DataFrame
df1
includes a new column 'Exists_in_DF2' that indicates whether each 'Key' element fromdf1
exists in the 'Ref' column ofdf2
.
Dynamic Membership Checks
Dynamically generate lists or Series to pass to the
isin()
method based on conditions or calculations.Apply these dynamic checks to DataFrames to manage data more robustly and flexibly.
pythonimport numpy as np df = pd.DataFrame({ 'Value': np.random.randint(1, 100, 10) }) range_values = np.arange(10, 51) # Creating a range from 10 to 50 df['InRange'] = df['Value'].isin(range_values) print(df)
In this example, the
isin()
method checks if each 'Value' in the DataFrame falls within the generated range from 10 to 50. The new column 'InRange' indicates this membership, making it easy to identify which values meet the condition.
Conclusion
Mastering the isin()
method in Pandas enhances your data manipulation capabilities significantly. This function's ability to handle checks against multiple data structures makes it indispensable for complex data analysis and filtering needs. Implementing this method helps streamline your data processing tasks, making data management tasks more manageable and your analyses more insightful and effective. Embrace this method to elevate the efficiency of your data-driven decision-making processes.
No comments yet.