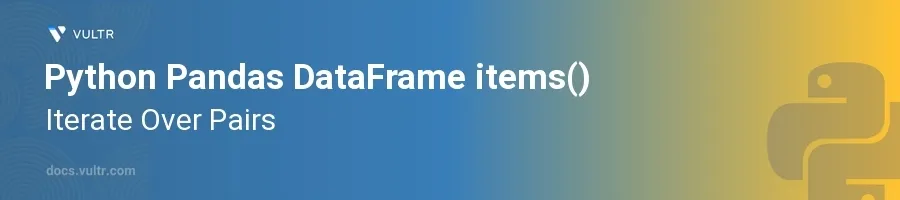
Introduction
The items()
method in Pandas is a versatile tool, crucial for iterating over DataFrame columns in a way that it yields each column as a pair of its corresponding label and content as a series. This method supports clear, concise, and efficient iteration over DataFrame pairs, which is essential for data analysis, manipulation, and cleansing tasks.
In this article, you will learn how to effectively use the items()
method in Python's Pandas library. Explore the method’s usefulness in handling DataFrame objects by demonstrating its application in various real-world data operations. Delve into examples that highlight how this method streamlines tasks involving data transformation and analysis.
Understanding the items() Method
Basic Usage of items()
Start with a simple DataFrame.
Use
items()
to iterate through the DataFrame to print each column name and data.pythonimport pandas as pd # Creating a sample DataFrame data = { 'Name': ['Alice', 'Bob', 'Charles'], 'Age': [25, 30, 35], 'Occupation': ['Engineer', 'Doctor', 'Artist'] } df = pd.DataFrame(data) # Using items() for label, content in df.items(): print(f"Column: {label}") print("Data:") print(content, "\n")
This example creates a DataFrame comprising
Name
,Age
, andOccupation
columns and iterates through each usingitems()
. For each iteration, both the column name (label) and the contents (series) are printed.
Working with Selected Data
Apply
items()
to conditionally process data columns.Utilize it to transform items that meet specific conditions in a DataFrame.
pythonimport pandas as pd df = pd.DataFrame({ 'Product': ['Tablet', 'Laptop', 'Desktop'], 'Price': [250, 800, 600] }) # Increasing prices by 10% where original price is over 500 for label, content in df.items(): if label == 'Price': df[label] = content.apply(lambda x: x * 1.10 if x > 500 else x) print(df)
In this code snippet, the
items()
method is used to manipulate the 'Price' column. For items costing over $500, a 10% price increase applies selectively, demonstrating conditional processing of data.
Advanced Data Manipulation with items()
Filtering Data
Use
items()
for more complex column-wise data filtering.Extract or modify data based on specific criteria.
pythonimport pandas as pd data = pd.DataFrame({ 'ID': [112, 113, 114], 'Value': [20, 40, 60], 'Status': ['pending', 'approved', 'denied'] }) # Filter and print rows where `Value` > 30 and `Status` is 'pending' or 'approved' filtered_data = {label: content for label, content in data.items() if label in ['Value', 'Status']} print(pd.DataFrame(filtered_data))
This snippet filters out the
Value
andStatus
columns and checks conditions, demonstrating a targeted approach to data examination and manipulation.
Transforming Data Based on Criteria
Enact transformations across several columns simultaneously.
Efficiently adjust data to fit analysis or output needs.
pythonimport pandas as pd df = pd.DataFrame({ 'Temperature': [22, 25, 19], 'Humidity': [80, 60, 77] }) # Convert temperature from Celsius to Fahrenheit and adjust humidity for label, content in df.items(): if label == 'Temperature': df[label] = content * 9 / 5 + 32 if label == 'Humidity': df[label] = content * 1.1 print(df)
Leveraging
items()
, this example converts temperatures from Celsius to Fahrenheit and scales the humidity readings by 10%. This showcases batch processing of data columns.
Conclusion
The items()
function in Python's Pandas module provides a robust way to iterate over DataFrame column pairs, offering clear advantages in analyzing and manipulating data effectively. Integrate this function into data processing routines to simplify tasks like conditional processing, data transformation, and complicated data filtering. By mastering these techniques, maintain clean, efficient, and highly readable data manipulation code. Whether performing simple or complex data operations, the items()
method is an indispensable tool in the arsenal of any data scientist or analyst.
No comments yet.