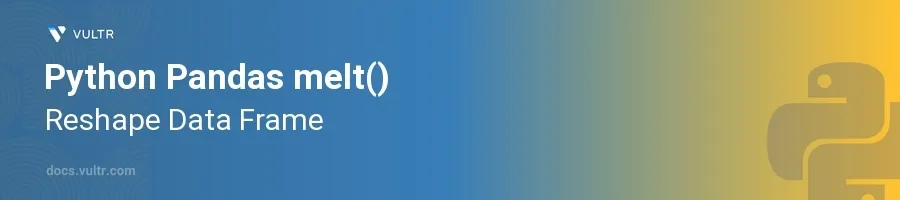
Introduction
When working with data in Python, the Pandas library provides a wide array of functionalities geared towards data manipulation and analysis. One such functionality is the melt()
function, which allows for the transformation of data frames from a wide format to a long format. This function is particularly useful in scenarios where you want to make your dataset compatible with other Python data analysis tools, or simply when you want to change the structure of your data for visualization purposes.
In this article, you will learn how to effectively use the melt()
function to reshape your data frames. Explore the fundamental concepts behind melting a data frame, various parameters of the melt()
function, and how to handle practical data reshaping tasks tailored for different scenarios.
Understanding the melt() Function
Before diving into examples, it’s crucial to grasp what the melt()
function does and how it can be utilized to reshape your data frames.
Basic Concepts and Parameters
The melt()
function is used to transform a data frame from a wide format (many columns) to a long format (few columns, but more rows). It essentially "melts" the data frame by turning columns into rows.
Recognize key parameters of
melt()
:id_vars
: Columns to use as identifier variables.value_vars
: Columns to melt/unpivot.var_name
: Name of the variable column in the melted data frame.value_name
: Name of the value column in the melted data frame.
Understand the structure change:
- Original data frames often have separate columns that are best represented as values under a single column.
melt()
reduces the number of columns by converting them into rows, enhancing the data frame structure for certain types of analyses.
Basic Usage Example
Let's start with a basic example to see melt()
in action.
Define a simple data frame:
pythonimport pandas as pd df = pd.DataFrame({ 'Day': ['Mon', 'Tue', 'Wed'], 'Apple': [1, 3, 5], 'Banana': [2, 4, 6] })
Apply the
melt()
function:pythonmelted_df = df.melt(id_vars=['Day'], value_vars=['Apple', 'Banana'], var_name='Fruit', value_name='Quantity') print(melted_df)
This shifts the 'Apple' and 'Banana' columns into a single 'Fruit' column, with their corresponding values under 'Quantity'. Each 'Day' now has two records, one for each fruit.
Advanced Usage Scenarios
Melting with Multiple Identifier Columns
Sometimes, datasets are more complex and need multiple identifiers to maintain data integrity during the melting process.
Consider a data frame with additional dimensions:
pythondf = pd.DataFrame({ 'Day': ['Mon', 'Tue', 'Wed'], 'Region': ['North', 'South', 'East'], 'Apple': [1, 3, 5], 'Banana': [2, 4, 6] })
Melt while preserving multiple identifiers:
pythonmelted_df = df.melt(id_vars=['Day', 'Region'], value_vars=['Apple', 'Banana'], var_name='Fruit', value_name='Quantity') print(melted_df)
Here, both 'Day' and 'Region' are used as identifiers, ensuring that each row in the melted data frame maintains a reference to both its day and geographical region.
Handling Missing Variables
In situations where not all columns are mentioned under value_vars
, Pandas automatically considers the rest of the columns as values to be melted.
Melt without specifying
value_vars
:pythondf2 = pd.DataFrame({ 'Day': ['Mon', 'Tue', 'Wed'], 'Apple': [1, 3, None], 'Banana': [2, None, 6] }) melted_df2 = df2.melt(id_vars='Day', var_name='Fruit', value_name='Quantity') print(melted_df2)
This snippet melts all columns except 'Day', treating them as variable columns with their respective values. It automatically handles
None
values as missing data in 'Quantity'.
Conclusion
Utilizing the melt()
function in Pandas effectively transforms your data frame from wide to long format, facilitating a variety of data analysis tasks. Whether dealing with simple or complex data structures, the melt()
function provides a robust way to reshape your data. Adjusting the parameters allows precise control over how the data is reshaped, making it a versatile tool in your data manipulation toolbox. By applying the techniques discussed, optimize data analysis and tailor the data structure precisely to your needs.
No comments yet.