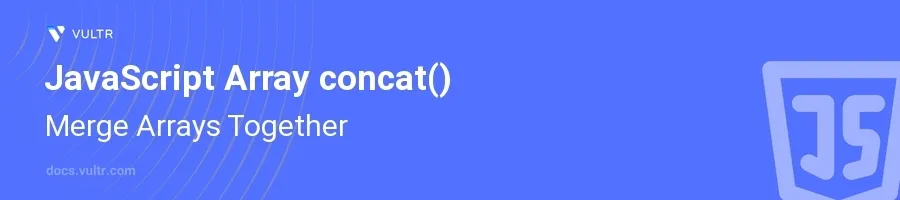
Introduction
The concat()
method in JavaScript is a critical tool for combining two or more arrays into a single array without altering the original arrays. This method provides a straightforward way to manage array data, particularly useful in scenarios involving data manipulation, aggregation, or when preparing data sets for further processing.
In this article, you will learn how to effectively use the concat()
method to merge multiple arrays. Explore practical examples that highlight how to combine arrays of primitive types, objects, and even how to handle nested arrays.
Using concat() with Basic Arrays
Merge Simple Arrays
Initialize two or more arrays with primitive data types.
Use the
concat()
method to combine these arrays into one.javascriptlet array1 = ['apple', 'banana']; let array2 = ['cherry', 'date']; let combinedArray = array1.concat(array2); console.log(combinedArray);
This code initializes two array variables
array1
andarray2
and merges them intocombinedArray
usingconcat()
. The resulting array is['apple', 'banana', 'cherry', 'date']
.
Concatenating Multiple Arrays
Understand that
concat()
can merge more than two arrays at a time.Provide multiple arrays as arguments to the
concat()
method.javascriptlet fruits = ['apple', 'banana']; let vegetables = ['carrot', 'beet']; let grains = ['wheat', 'rice']; let combinedFood = fruits.concat(vegetables, grains); console.log(combinedFood);
Here, three arrays
fruits
,vegetables
, andgrains
are merged into a single large arraycombinedFood
. The method streamlines the merging of multiple collections efficiently.
Using concat() with Complex Structures
Merging Arrays of Objects
Define arrays containing object literals.
Merge these arrays while noting that objects remain independent in memory, maintaining their distinct references.
javascriptlet array1 = [{ name: 'John' }, { name: 'Jane' }]; let array2 = [{ age: 22 }, { age: 23 }]; let combinedDetails = array1.concat(array2); console.log(combinedDetails);
This script merges two arrays of objects. Notice that each object in the resulting
combinedDetails
array maintains its structure and reference, illustratingconcat()
versatility in handling complex data types.
Handling Nested Arrays
Recognize that
concat()
does not flatten arrays but preserves their structure.Test this by merging arrays that have nested arrays within them.
javascriptlet nested1 = [1, 2, [3, 4]]; let nested2 = [5, [6, 7]]; let combinedNested = nested1.concat(nested2); console.log(combinedNested);
The output of this example shows that nested arrays are preserved in the structure of the merged array. The resulting array is
[1, 2, [3, 4], 5, [6, 7]]
, clearly demonstrating the non-flattening nature of theconcat()
method.
Conclusion
The concat()
function in JavaScript is a potent tool for merging arrays, suitable for handling both simple and complex data structures. Its use extends from combining lists of primitive types to merging more intricate objects and even nested arrays. By using the techniques discussed, you empower your JavaScript projects with efficient and readable array manipulations. Whether working on web applications or data processing tasks, understanding how to leverage concat()
enhances your capability to manage array-based data effectively.
No comments yet.