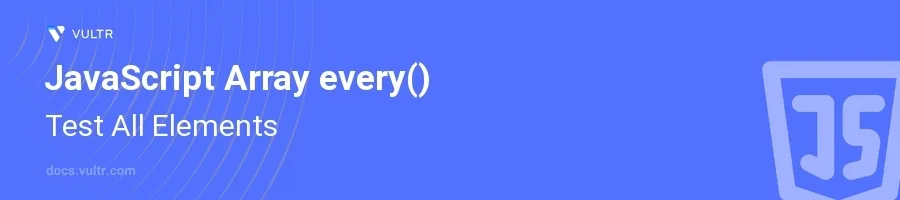
Introduction
The every()
method in JavaScript is a high-order function, particularly useful for validating all elements in an array against a specific condition. Employed mainly for ensuring that every item in a JavaScript array passes a particular test, this method is essential when you need uniformity across array elements. It returns a Boolean value, making it highly effective for checks that require a straightforward true or false decision.
In this article, you will learn how to utilize the every()
method in JavaScript for various practical applications. Explore how this method ensures all elements in a JavaScript array meet a set criterion, using clear examples that demonstrate its usage in day-to-day coding tasks.
Understanding the JavaScript every() Method
Basic Usage of every()
Start with defining an array.
Use the
every()
method and pass a test function to it.javascriptconst numbers = [10, 20, 30, 40, 50]; const isAboveNine = numbers.every(number => number > 9); console.log(isAboveNine);
In this code, the
every()
method evaluates whether each element in thenumbers
array is greater than 9. Since all elements meet this criterion,every()
returnstrue
.
Validating All Elements for a Specific Condition
Prepare an array with object elements.
Invoke the
every()
method with a condition to check a specific property.javascriptconst users = [ { name: "Alice", age: 25 }, { name: "Bob", age: 34 }, { name: "Charlie", age: 47 } ]; const areAllAdults = users.every(user => user.age >= 18); console.log(areAllAdults);
Above,
every()
checks if theage
property of each user object in the array satisfies the adulthood condition (being at least 18 years old). In this case, the result istrue
.
Using JavaScript every() Method to Test Data Types and Content
Check for Uniform Data Types
Define an array containing different types of data.
Apply the
every()
method to test if all items are of the same type.javascriptconst mixedArray = [1, 'text', true, 20]; const allNumbers = mixedArray.every(item => typeof item === 'number'); console.log(allNumbers);
The function checks if every item in
mixedArray
is a number. AsmixedArray
includes various types, the function returnsfalse
.
Verifying the Presence of Custom Conditions
Create an array to hold a complex condition.
Use the
every()
method to verify this condition.javascriptconst values = [2, 4, 6, 8, 10]; const allEven = values.every(value => value % 2 === 0); console.log(allEven);
This snippet checks if every number in the
values
array is even. As all elements satisfy this condition, the result istrue
.
Conclusion
The every()
method in JavaScript provides an efficient solution for ensuring that every element in an array satisfies a specific condition. By returning a Boolean value, it offers a clear, binary response, making it ideal for various tests, such as type-checking or more complex conditional evaluations. Implement the every()
method in your next project to enforce consistency and correctness in data handling and decision-making processes. Through the examples provided, streamline your coding efforts by using this efficient and effective array method.
No comments yet.