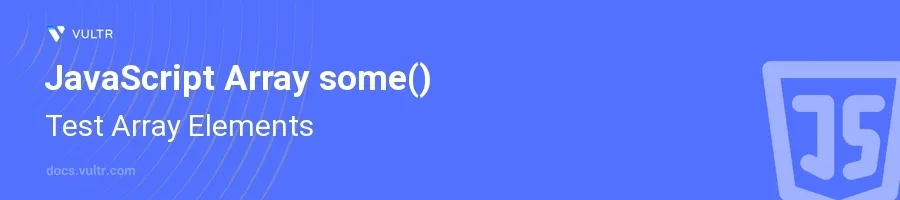
Introduction
The some()
method in JavaScript provides a concise and efficient way to test at least one element of an array against a specified condition. This array method is particularly useful in scenarios where you need to check if any elements in an array meet certain criteria, such as validating user inputs or filtering data based on specific conditions.
In this article, you will learn how to wield the some()
method in various use cases. Understand its utility in operations that involve arrays while exploring how it differs from similar methods and discovering best practices for incorporating some()
in your JavaScript projects.
Understanding the some() Method
Basic Usage of some()
Define an array of elements.
Pass a callback function to
some()
that specifies the condition to test on array elements.javascriptconst numbers = [1, 2, 3, 4, 5]; const hasEven = numbers.some(number => number % 2 === 0); console.log(hasEven);
This code checks if there is at least one even number in the
numbers
array. Thesome()
method returnstrue
in this case since2
and4
are even.
Using some() with an Array of Objects
Create an array of objects where each object represents a distinct entity with various properties.
Use
some()
to determine if any object meets a particular condition.javascriptconst people = [ { name: "Alice", age: 25 }, { name: "Bob", age: 17 }, { name: "Charlie", age: 30 } ]; const hasMinors = people.some(person => person.age < 18); console.log(hasMinors);
In this snippet,
some()
checks if any person in the array is a minor (under 18 years old). It returnstrue
because "Bob" is 17 years old.
Practical Applications of some()
Validation Scenarios
Consider an array storing input values from a form.
Use
some()
to quickly validate if any of the inputs are empty or invalid.javascriptconst inputs = ["username", "", "email@example.com"]; const isEmpty = inputs.some(input => input === ""); console.log(isEmpty);
Here,
some()
validates the inputs and returnstrue
because one of the inputs is an empty string, indicating a potentially invalid form submission.
Integration with Promises
Use
some()
in asynchronous operations, especially when dealing with arrays of Promises or data fetched in parallel.Combine
some()
withasync/await
to handle specific logic based on the presence or absence of certain conditions.javascriptasync function checkOnlineStatus(users) { const promises = users.map(user => fetch(`/status/${user.id}`).then(res => res.json())); const results = await Promise.all(promises); const isAnyoneOnline = results.some(status => status.online); console.log(isAnyoneOnline); }
The
some()
method here helps determine if any user in a list fetched asynchronously is currently online.
Conclusion
The some()
function in JavaScript serves as a powerful utility for testing whether any elements in an array satisfy a given condition. Its ability to streamline the evaluation of array elements makes it an indispensable tool for scenarios requiring conditional checking, such as form validation or data filtering. By utilizing the techniques discussed, you can ensure your JavaScript code is not only efficient but also robust and maintainable.
No comments yet.